How to create database tables with Entity Framework Code-First?
Creating database tables with Entity Framework Code-First involves defining domain classes, configuring their mappings, and then executing migrations to generate the corresponding database schema. Here’s a step-by-step guide on how to create database tables using Entity Framework Code-First:
- Define Domain Classes: Start by creating C# classes that represent the tables you want in your database. Each class should define properties that correspond to table columns. For example, if you’re creating a “Product” table, you might have a `Product` class with properties like `ProductId`, `Name`, `Price`, etc.
- Create a DbContext: Create a class that derives from `DbContext`. This class acts as the entry point for Entity Framework to interact with the database. In your `DbContext` class, define a `DbSet` property for each domain class you want to map to a table. For example:
```csharp public class ApplicationDbContext : DbContext { public DbSet<Product> Products { get; set; } } ```
- Configuration (Optional): If you need to customize the database schema, you can use Fluent API or data annotations to specify things like table names, primary keys, relationships, and column constraints. This step is optional, as Entity Framework can infer the schema from your class definitions.
- Enable Migrations: Open the Package Manager Console in Visual Studio and run the following command to enable migrations:
```powershell Enable-Migrations ```
5. Add Initial Migration: Run the following command to scaffold an initial migration:
```powershell Add-Migration InitialCreate ```
- Update Database: Finally, apply the migration to create the database tables:
```powershell Update-Database ```
Entity Framework will generate the database tables based on your domain classes and configurations. If you make changes to your domain classes later on, you can create new migrations using `Add-Migration` and update the database using `Update-Database` again to reflect those changes in the database schema.
By following these steps, you can effectively create database tables using Entity Framework Code-First, making it easier to manage your database schema alongside your C# application code.
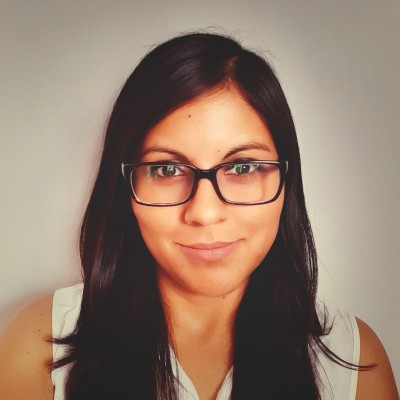
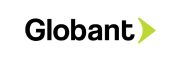