How to disable lazy loading in Entity Framework?
Disabling Lazy Loading in Entity Framework is a straightforward process, and it can be useful in scenarios where you want to have more control over when and how related data is loaded. Lazy Loading is enabled by default, but you can disable it at the context level or the entity level, depending on your requirements.
- Disable Lazy Loading at the Context Level:
To disable Lazy Loading for all entities within a specific `DbContext`, you can set the `LazyLoadingEnabled` property to `false`. Here’s an example:
```csharp public class MyDbContext : DbContext { public MyDbContext() { // Disable Lazy Loading Configuration.LazyLoadingEnabled = false; } // DbSet properties and other configurations } ```
By setting `LazyLoadingEnabled` to `false` in the constructor of your `DbContext`, you ensure that Lazy Loading is turned off for all entities managed by that context.
- Disable Lazy Loading for Specific Entities:
If you want to disable Lazy Loading for specific entities while keeping it enabled for others, you can use the `virtual` keyword on navigation properties and then use the `ProxyCreationEnabled` property to control Lazy Loading. Here’s an example:
```csharp public class Order { public int OrderId { get; set; } public string OrderNumber { get; set; } // Disable Lazy Loading for OrderDetails public virtual ICollection<OrderDetail> OrderDetails { get; set; } } public class MyDbContext : DbContext { public MyDbContext() { // Disable Lazy Loading for specific entity Configuration.ProxyCreationEnabled = false; } // DbSet properties and other configurations } ```
In this case, setting `ProxyCreationEnabled` to `false` at the context level will disable Lazy Loading only for entities that have navigation properties marked as `virtual`.
Disabling Lazy Loading can be beneficial when you want to optimize performance, especially in scenarios where you need to load related data in a more controlled and predictable manner using techniques like eager loading (`.Include()`) or explicit loading (`.Load()`). It allows you to have more control over how and when related data is fetched from the database.
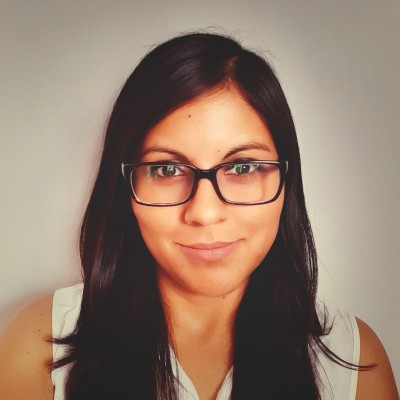
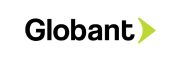