Unlocking Efficiency and Performance through Effective Multithreading Techniques in C#
As computer systems continue to evolve, the potential for using their processing capabilities to the fullest expands correspondingly. One of the ways to leverage this power, often applied by skilled C# developers, is by making use of multithreading in programming. This article will focus on multithreading in the C# programming language, delving into the fundamental concepts of concurrent programming, its advantages, and how it can be used effectively when you hire C# developers who understand these concepts deeply.
What is Multithreading?
Multithreading is a prevalent feature of modern programming. It’s a form of concurrent execution of programs in which a single process is divided into two or more sub-processes that can run simultaneously, sharing the system’s resources. This concept is based on threads, which are the smallest units of a process that can execute independently. Each thread has its stack and local variables.
In C#, a single-threaded application operates sequentially, completing one task before starting another. However, a multi-threaded application can handle several tasks concurrently, providing enhanced performance, particularly in scenarios where the application needs to manage a large number of user requests or perform complex calculations.
Multithreading in C#
C# provides rich built-in features and libraries for multithreading, which forms an integral part of .NET’s System.Threading namespace. Developers can create, start, pause, and stop threads in a flexible manner, allowing for efficient utilization of the system’s CPU resources.
To create a new thread in C#, you’ll typically define a new `Thread` object, passing the method to run as a thread to the Thread constructor. This method represents the thread’s behavior when it’s executed.
Here’s a basic example:
```csharp Thread newThread = new Thread( new ThreadStart(MethodName)); newThread.Start();
The Advantages of Multithreading
Multithreading offers several key advantages:
- Improved performance: Since threads run concurrently, programs can accomplish more in less time, making the most of the CPU resources.
- Better responsiveness: In a GUI application, a separate thread can be used for UI, while other threads handle background tasks, improving the user experience by preventing the application from becoming unresponsive.
- Simplified modeling: In some scenarios, using multiple threads can make the design of the program more straightforward.
Despite its advantages, multithreading also comes with its complexities, such as the need for synchronization and the potential for deadlock situations. It’s important to understand these concepts before delving deeper into multithreading.
Thread Synchronization
When multiple threads are manipulating the same data concurrently, the program might display erratic behavior due to the inconsistency of the shared data. This problem is known as a race condition. To avoid race conditions, it’s necessary to ensure that the shared data is accessed mutually exclusively by threads. This concept is known as thread synchronization.
In C# The `lock` keyword is commonly used for synchronization. It restricts the marked code so that it can only be executed by one thread at a time. Here’s a simple example:
```csharp private Object lockObj = new Object(); lock (lockObj) { // Code to be executed by one thread at a time. }
Deadlocks
A deadlock is a state often encountered in complex multithreading scenarios where two or more threads are unable to proceed because each is waiting for the other to release a resource. When you hire C# developers with a deep understanding of these challenges, they’re well-equipped to handle such situations. Deadlocks can cause an application to hang and are tricky to debug and prevent, making it crucial for your C# developers to have thorough knowledge in this area. They occur in situations where a circular wait is present, meaning that each thread is waiting for a resource that the other threads are holding. By hiring experienced C# developers, you can ensure that your software avoids these pitfalls and runs smoothly and efficiently.
Here’s a simple example to illustrate a deadlock scenario:
Consider two threads, `Thread1` and `Thread2`, and two resources, `ResourceA` and `ResourceB`.
- `Thread1` is holding `ResourceA` and requests `ResourceB`.
- At the same time, `Thread2` is holding `ResourceB` and requests `ResourceA`.
Now, neither of the threads can proceed. `Thread1` can’t get `ResourceB` because it’s held by `Thread2`, and `Thread2` can’t get `ResourceA` because it’s held by `Thread1`. Both threads are in a state of waiting for each other to release a resource, and hence, a deadlock occurs.
In C# code, this could look something like this:
```csharp object lock1 = new object(); object lock2 = new object(); void Thread1() { lock (lock1) { Thread.Sleep(1000); lock (lock2) { Console.WriteLine("Thread1"); } } } void Thread2() { lock (lock2) { Thread.Sleep(1000); lock (lock1) { Console.WriteLine("Thread2"); } } }
In this example, `Thread1` acquires `lock1` and `Thread2` acquires `lock2`. Both then sleep for a second, after which they try to acquire the other lock. But since they are each held by the other thread, neither can proceed, resulting in a deadlock.
To avoid deadlocks, developers must be careful about the order in which resources are locked and released, and they should avoid nested locks whenever possible.
Advanced Multithreading in C#: Tasks and the Task Parallel Library
While lower-level thread manipulation is certainly possible in C#, a more convenient and powerful way to handle multithreading is through the Task Parallel Library (TPL). This library offers high-level approaches to parallel programming by introducing the concept of a Task, which represents an asynchronous operation. Tasks are easier to compose and coordinate than raw threads, and they provide more control over parallel execution.
Here’s a simple task creation example:
```csharp Task myTask = Task.Factory.StartNew(() => { // Code to be executed by the task. }); myTask.Wait();
The TPL also includes features for handling exceptions, canceling tasks, and more complex scenarios such as parallel loops and data parallelism.
Conclusion
Multithreading in C# is a powerful feature that can significantly enhance the performance and responsiveness of applications. It introduces complexity, particularly with thread synchronization and the potential for deadlocks. However, when you hire C# developers with a solid understanding of these aspects, they can leverage robust tools and libraries like the Task Parallel Library provided by C# to simplify these challenges. By effectively utilizing these features, C# developers can unlock the full potential of concurrent programming. As always with powerful tools, multithreading must be handled carefully. When managed poorly, it can lead to unexpected results. However, when you hire C# developers who can manage it well, it serves as a key to creating higher-performing, more efficient, and responsive applications.
Table of Contents
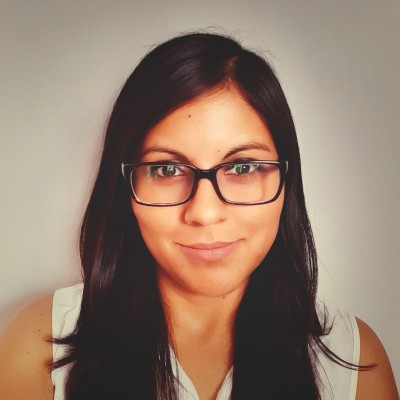
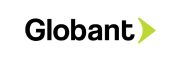