What is encapsulation in C#?
Encapsulation is one of the fundamental principles of object-oriented programming (OOP) and plays a crucial role in C# programming. It refers to the practice of bundling data (attributes or properties) and the methods (functions or behaviors) that operate on that data into a single unit known as a class. Encapsulation provides a way to hide the internal state of an object and restrict direct access to its data, allowing controlled and secure interaction with the object.
In C#, encapsulation is achieved through access modifiers like `public`, `private`, `protected`, and `internal`. These modifiers control the visibility and accessibility of class members (fields, properties, and methods). Here’s a breakdown of how encapsulation works:
- Private Data: Class fields and properties are typically declared as `private` to restrict direct access from outside the class. This means that the internal state of an object is hidden from external code.
- Public Interfaces: Public methods and properties are provided to access and modify the encapsulated data. These methods act as gatekeepers, ensuring that data is accessed and modified in a controlled and validated manner.
- Getters and Setters: Properties in C# often include both a getter and a setter. The getter allows reading the data, while the setter enables modifying it. This provides fine-grained control over data access.
Here’s a simplified example:
```csharp public class BankAccount { private decimal balance; public decimal Balance { get { return balance; } private set { balance = value; } } public BankAccount(decimal initialBalance) { Balance = initialBalance; } public void Deposit(decimal amount) { if (amount > 0) Balance += amount; } public void Withdraw(decimal amount) { if (amount > 0 && amount <= Balance) Balance -= amount; } } ```
In this example, the `balance` field is encapsulated within the `BankAccount` class. The `Balance` property provides controlled access to the balance, ensuring that it can only be modified through the `Deposit` and `Withdraw` methods. Encapsulation promotes data integrity, security, and maintainability by hiding the internal details of a class and allowing controlled access to its state. It is a fundamental concept for building robust and reliable C# applications.
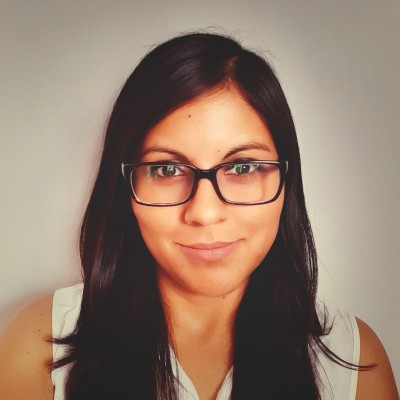
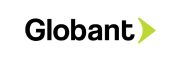