C# Q & A
How to use Entity Framework Core for database access in C#?
Using Entity Framework Core (EF Core) for database access in C# is a straightforward and efficient way to interact with relational databases. Here’s a step-by-step guide on how to use EF Core for database access:
- Install EF Core: Start by installing Entity Framework Core using NuGet Package Manager in your C# project. You’ll need the `Microsoft.EntityFrameworkCore` package, and if you’re working with a specific database provider (e.g., SQL Server, SQLite, PostgreSQL), you’ll also need the corresponding provider package (e.g., `Microsoft.EntityFrameworkCore.SqlServer`).
- Create DbContext: In your C# project, define a class that inherits from `DbContext`. This class represents your database context and serves as a bridge between your application and the database. In this class, use DbSet properties to define your entity types, which correspond to database tables.
- Configure Database Connection: Configure your database connection string in your application’s configuration settings. You can use the `DbContextOptions` to specify the database provider and connection string in your DbContext class.
- Define Entity Classes: Create C# classes that represent your database tables. Annotate these classes with attributes or Fluent API configurations to define relationships, primary keys, and other database-specific details.
- CRUD Operations: Use LINQ queries and methods provided by EF Core to perform CRUD (Create, Read, Update, Delete) operations on your entities. You can use methods like `Add`, `Find`, `Update`, and `Remove` to manipulate data.
- Migrations: EF Core provides a migration mechanism to create and update the database schema based on your entity classes. Use the `dotnet ef` command-line tool to create and apply migrations to keep your database schema in sync with your code.
- Error Handling: Implement proper error handling and validation when working with EF Core to handle exceptions and database-related errors gracefully.
- Dependency Injection: In your application’s startup configuration, set up dependency injection for your DbContext. This allows you to inject your database context into your application services and components, promoting modularity and testability.
- Testing: Write unit tests for your database access code to ensure that your data operations work as expected. EF Core’s in-memory database provider is a helpful tool for testing without touching a real database.
- Optimization: As your application grows, consider optimizing database queries and data access patterns to improve performance. EF Core offers various features like eager loading, lazy loading, and query optimization to help with this.
Entity Framework Core simplifies database access in C# by providing a high-level, object-oriented approach to interacting with databases. It abstracts many of the complexities of data access, allowing developers to focus on application logic while seamlessly integrating with various database providers.
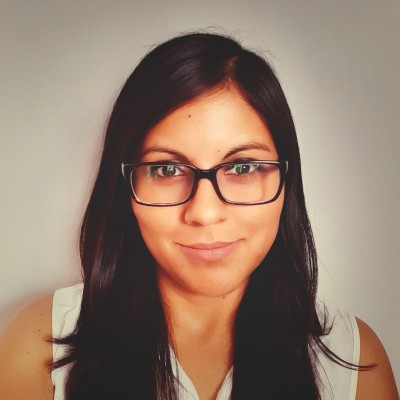
Previously at
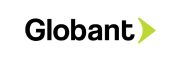
Experienced Backend Developer with 6 years of experience in C#. Proficient in C#, .NET, and Java.Proficient in REST web services and web app development.