What is Entity Framework Core Transactions in C#?
Entity Framework Core (EF Core) transactions in C# provide a way to manage database operations as a single, atomic unit of work. Transactions ensure that a series of database operations either succeed entirely or fail entirely, maintaining data integrity and consistency. They are crucial when dealing with situations where multiple operations must be coordinated and treated as a single operation.
In EF Core, you can work with transactions using the `DbContext` class. Here’s how it typically works:
- Begin a Transaction: You start by creating a transaction scope using the `BeginTransaction` method of your `DbContext`:
```csharp using var dbContext = new YourDbContext(); using var transaction = dbContext.Database.BeginTransaction(); ```
- Perform Database Operations: Within the transaction scope, you can execute various database operations like inserts, updates, or deletes:
```csharp dbContext.Add(entity1); dbContext.Update(entity2); dbContext.Remove(entity3); ```
- Commit or Rollback: After executing the operations, you have two options:
– Commit: If everything goes well and you want to persist the changes, you can call `transaction.Commit()`. This makes all changes permanent in the database.
– Rollback: If an error occurs or you decide to discard the changes, you can call `transaction.Rollback()`. This undoes all changes made within the transaction.
Here’s a sample code snippet:
```csharp using var dbContext = new YourDbContext(); using var transaction = dbContext.Database.BeginTransaction(); try { // Perform database operations dbContext.Add(entity1); dbContext.Update(entity2); // Commit the transaction transaction.Commit(); } catch (Exception) { // Handle exceptions and roll back if necessary transaction.Rollback(); } ```
Transactions are essential for maintaining data consistency, especially in scenarios where multiple related database operations need to be coordinated. They ensure that either all changes are applied successfully, or none of them are, preventing data corruption or incomplete updates.
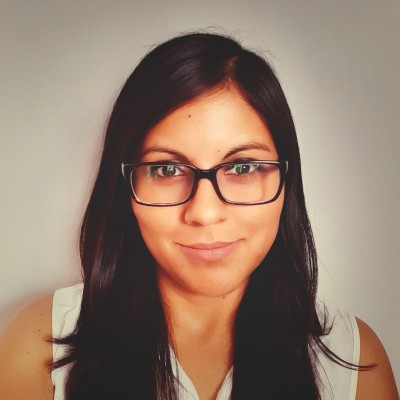
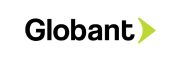