How to use Entity Framework for database access in C#?
Using Entity Framework (EF) for database access in C# involves several steps and best practices to ensure efficient and reliable data operations. Here’s a concise guide on how to utilize EF for database access:
- Install Entity Framework:
Start by installing Entity Framework through NuGet Package Manager in Visual Studio. You can install the Entity Framework package specific to your database provider (e.g., EntityFramework.SqlServer for SQL Server).
- Create a Data Model:
Define your data model by creating C# classes that represent database tables. Use attributes like `[Key]`, `[Required]`, and `[ForeignKey]` to specify primary keys, required fields, and relationships between entities.
- DbContext Configuration:
Create a class that inherits from `DbContext`, which serves as the main entry point for interacting with your database. In the constructor of your `DbContext` class, specify the database connection string.
- CRUD Operations:
Use your `DbContext` instance to perform CRUD (Create, Read, Update, Delete) operations on your data model. For example, to retrieve data, you can use LINQ queries or methods like `Find` or `FirstOrDefault`. For inserts and updates, create new instances of your model classes and use `Add` and `Update` methods.
- Save Changes:
After making changes to your entities, call the `SaveChanges` method on your `DbContext` instance to persist the changes to the database. This should be done within a transaction, and error handling should be in place to handle any exceptions.
- Using Asynchronous Programming:
Consider using asynchronous methods (e.g., `SaveChangesAsync`, `ToListAsync`) to improve the responsiveness of your application, especially in scenarios with high concurrency or long-running operations.
- Error Handling and Validation:
Implement error handling and validation for your database operations. Entity Framework provides mechanisms for validation, and you can use `try-catch` blocks to handle exceptions gracefully.
- Database Migrations:
If you’re using the Code-First approach, create and apply database migrations to keep the database schema in sync with your data model changes. Use the `Add-Migration` and `Update-Database` commands in the Package Manager Console.
- Logging and Monitoring:
Consider integrating logging and monitoring tools to track database-related issues, optimize queries, and identify performance bottlenecks.
- Security and Permissions:
Implement appropriate security measures, such as role-based access control (RBAC) and parameterized queries, to protect against SQL injection and unauthorized access.
By following these steps and best practices, you can effectively use Entity Framework for database access in C#. EF abstracts many of the complexities of working with databases, making it a powerful tool for data-driven applications while promoting maintainability and robustness in your codebase.
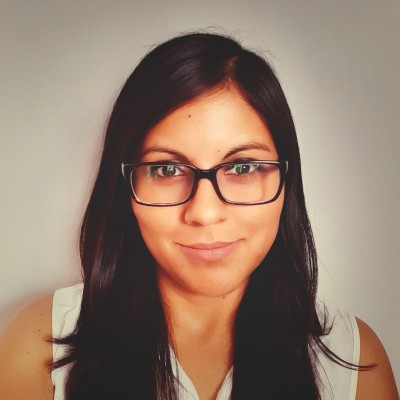
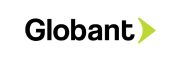