What is Entity Framework lazy loading in C#?
Entity Framework (EF) Lazy Loading is a feature that enhances the efficiency of data retrieval in your C# applications by loading related data only when it’s explicitly accessed. It is a valuable aspect of Entity Framework’s approach to handling object-relational mapping (ORM) and helps reduce the amount of data fetched from the database until it’s actually needed.
Here’s how EF Lazy Loading works:
- Deferred Loading: When you query the database for an entity (e.g., a record in a table), Entity Framework retrieves only that entity’s data initially. Related entities, such as navigation properties or child entities, are not loaded from the database at this stage.
- On-Demand Loading: Lazy Loading allows related data to be loaded on-demand. This means that when you access a navigation property, EF will transparently issue a query to the database to retrieve the associated data.
For example, consider a scenario where you have an `Order` entity with a navigation property `OrderDetails`. With Lazy Loading enabled, accessing `order.OrderDetails` will trigger a database query to fetch the order details only when you first access this property.
To enable Lazy Loading in Entity Framework, you typically use the `virtual` keyword on navigation properties. Here’s an example:
```csharp public class Order { public int OrderId { get; set; } public string OrderNumber { get; set; } // Enable Lazy Loading for OrderDetails public virtual ICollection<OrderDetail> OrderDetails { get; set; } } ```
It’s important to note that while Lazy Loading can be convenient, it should be used judiciously. Excessive Lazy Loading can lead to the infamous “N+1 query” problem, where multiple queries are executed to load related data, potentially impacting performance. To mitigate this, consider using techniques like eager loading (`.Include()`), explicit loading, or disabling Lazy Loading when it’s not needed for a particular scenario.
Entity Framework Lazy Loading in C# is a mechanism that defers the loading of related data until it’s accessed, helping improve application performance by reducing unnecessary database queries. It’s a valuable feature to optimize data retrieval in Entity Framework-powered applications.
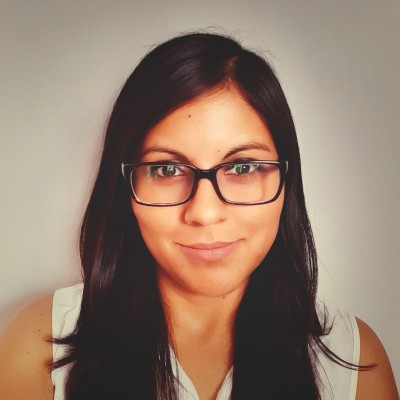
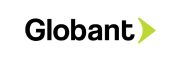