C# Q & A
How to generate models from an existing database with Entity Framework Database-First?
To generate models from an existing database using Entity Framework Database-First, you can follow these steps:
- Create a New Project: Start by creating a new C# project in your preferred development environment, such as Visual Studio.
- Install Entity Framework: If you haven’t already, install Entity Framework (EF) into your project using NuGet Package Manager. You can do this by right-clicking on your project, selecting “Manage NuGet Packages,” and searching for “Entity Framework.”
- Add a Connection String: Open your project’s configuration file (usually `app.config` or `web.config`) and add a connection string that points to your existing database. This connection string should specify the database provider (e.g., SQL Server, MySQL) and connection details (server name, database name, authentication credentials).
```xml <connectionStrings> <add name="YourDbContextName" connectionString="Data Source=ServerName;Initial Catalog=DatabaseName;User Id=Username;Password=Password;" providerName="System.Data.SqlClient" /> </connectionStrings> ```
- Generate Models: With the connection string in place, you can now use the Entity Framework’s scaffolding tools to generate models from the existing database. Open the Package Manager Console and run the following command:
```powershell Scaffold-DbContext "YourConnectionString" Microsoft.EntityFrameworkCore.SqlServer -OutputDir Models ```
Replace `”YourConnectionString”` with the name of the connection string you added earlier. The `-OutputDir` parameter specifies where the generated models will be placed. In this example, they will be placed in a “Models” folder.
- Customize Generated Models (Optional): After generating the models, you can customize them as needed. Entity Framework creates classes that map to your database tables, and you can add additional properties, methods, or attributes to these classes to suit your application’s requirements.
- Use DbContext: Now that you have the generated models, you can use the automatically generated DbContext class to query and manipulate data in your C# application. The DbContext will include DbSet properties for each table in your database.
By following these steps, you can quickly generate models from an existing database using Entity Framework Database-First. This approach saves you time and effort by automatically creating the necessary C# classes to work with your database schema.
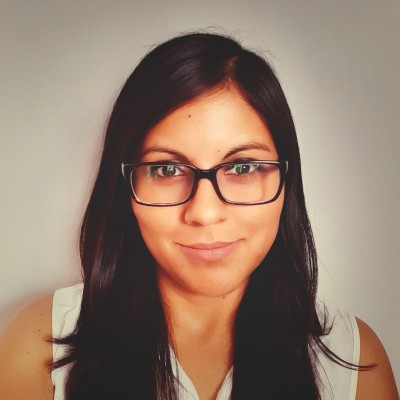
Previously at
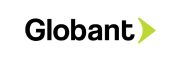
Experienced Backend Developer with 6 years of experience in C#. Proficient in C#, .NET, and Java.Proficient in REST web services and web app development.