What is inheritance in C#?
Inheritance is a fundamental concept in object-oriented programming (OOP), and it plays a significant role in C# as well. Inheritance allows you to create a new class (the derived class or subclass) based on an existing class (the base class or superclass). The derived class inherits the properties and methods of the base class, enabling code reuse and the creation of a hierarchy of related classes.
The key idea behind inheritance is the “is-a” relationship, which means that the derived class is a specialized version of the base class. This relationship allows you to model real-world scenarios effectively. For example, you can have a base class `Vehicle` with common properties like `Speed` and `Capacity`, and then create derived classes like `Car` and `Truck` that inherit these properties and add their own specific attributes or methods.
In C#, inheritance is established using the `:` symbol, followed by the name of the base class. Here’s a simplified example:
```csharp public class Vehicle { public int Speed { get; set; } public int Capacity { get; set; } } public class Car : Vehicle { public bool IsConvertible { get; set; } } ```
In this example, the `Car` class inherits the `Speed` and `Capacity` properties from the `Vehicle` class. It can also have additional properties like `IsConvertible`. By doing so, you avoid duplicating code and promote a hierarchical structure that simplifies code maintenance and enhances code readability.
Inheritance in C# allows you to create new classes by building upon existing ones, promoting code reuse and the modeling of real-world relationships. It’s a powerful OOP concept that helps you create more organized and maintainable code, especially when dealing with complex class hierarchies.
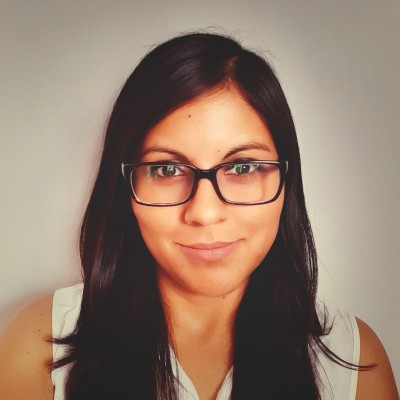
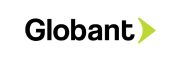