How to create an instance of a class in C#?
In C#, creating an instance of a class is a fundamental process that involves using the class as a blueprint to generate individual objects. Each object, or instance, has its own set of attributes (fields and properties) and behaviors (methods) defined by the class. Here’s how you create an instance of a class in C#:
- Identify the Class: Begin by identifying the class you want to instantiate. Classes serve as templates for objects, defining their structure and behavior. For example, if you have a class named `Person`, you’ll use this class to create instances of people.
- Use the `new` Keyword: To create an instance of a class, you use the `new` keyword followed by the class name and parentheses. For example:
```csharp Person person1 = new Person(); ```
This statement creates an instance of the `Person` class and assigns it to the variable `person1`.
- Optional Constructor Parameters: If the class has one or more constructors, you may need to provide values for any required parameters. Constructors are special methods responsible for initializing objects. For instance:
```csharp Person person2 = new Person("Alice", 25); ```
Here, we’re creating a `Person` object with the name “Alice” and age 25 by using a parameterized constructor.
- Access Object Members: Once an instance is created, you can access its members, such as fields, properties, and methods, using the dot notation. For example:
```csharp Console.WriteLine(person1.Name); // Accessing a property person2.SayHello(); // Calling a method ```
Here, we access the `Name` property of `person1` and call the `SayHello` method on `person2`.
Creating instances of classes in C# is a fundamental concept, and it allows you to work with data and behaviors defined within the class. By following these steps, you can create objects based on your class definitions and leverage the power of object-oriented programming in your C# applications.
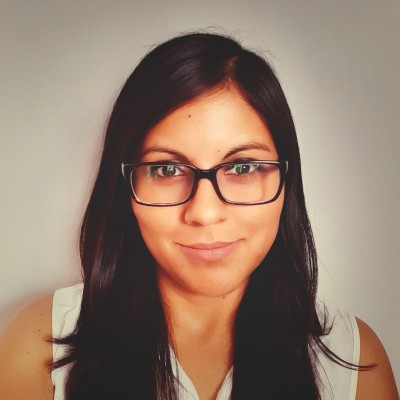
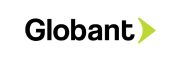