What is a C# interface?
In C#, an interface is a fundamental concept of object-oriented programming (OOP) that defines a contract or a set of method and property signatures that a class must adhere to if it implements the interface. An interface specifies what methods and properties a class should provide without specifying the actual implementation. It acts as a blueprint for a certain behavior, ensuring that classes that implement the interface provide specific functionality.
Key characteristics of C# interfaces include:
- Method and Property Signatures: An interface defines method and property signatures that participating classes must implement. These signatures include the method name, return type, and parameter list but do not contain any method body or implementation details.
- Implements Keyword: To indicate that a class adheres to an interface, it must use the `implements` keyword and provide implementations for all the methods and properties defined by the interface.
- Multiple Interface Implementation: A class in C# can implement multiple interfaces, allowing it to inherit and provide functionality from multiple sources.
- Achieving Polymorphism: Interfaces enable polymorphism, allowing objects of different classes to be treated as objects of the interface type. This promotes code reusability and flexibility.
Here’s a simplified example of an interface and its implementation:
```csharp // Define an interface public interface IShape { double CalculateArea(); } // Implement the interface in a class public class Circle : IShape { private double radius; public Circle(double r) { radius = r; } public double CalculateArea() { return Math.PI * Math.Pow(radius, 2); } } ```
In this example, the `IShape` interface defines the `CalculateArea` method signature, and the `Circle` class implements this interface by providing an implementation for `CalculateArea`. This allows instances of `Circle` to be treated as objects of type `IShape`, promoting code flexibility and adherence to the specified contract.
Interfaces are a fundamental building block in C# programming, enabling abstraction, code organization, and the definition of contracts that classes must follow, ensuring consistent behavior across different implementations.
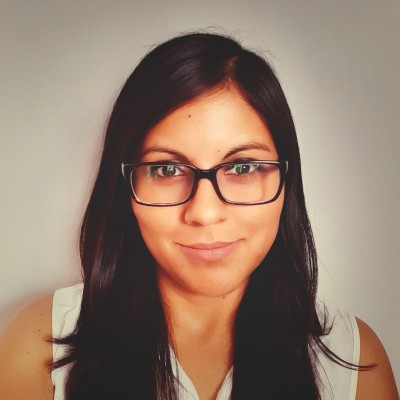
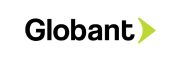