How to optimize database queries in Entity Framework?
Optimizing database queries in Entity Framework (EF) is essential for ensuring that your application performs efficiently and responds quickly to user requests. Here are several strategies to help you optimize your database queries in Entity Framework:
- Use Proper Indexing:
– Analyze your database schema and identify the columns frequently used in WHERE clauses or JOIN conditions.
– Ensure that these columns are indexed appropriately. Indexes can significantly speed up query performance.
- Lazy Loading vs. Eager Loading:
– Entity Framework supports both lazy loading and eager loading. Lazy loading can lead to the N+1 query problem, where separate queries are executed for related entities.
– Eager loading allows you to fetch related data in a single query using the `Include` method or projection queries with `Select`.
- Projection Queries:
– Instead of retrieving entire entities, use projection queries with the `Select` method to fetch only the required fields. This reduces the amount of data transferred from the database.
- Batching and Pagination:
– Implement pagination and batch fetching to limit the number of records returned in a single query. This prevents overloading your application with too much data.
- Avoid N+1 Query Problem:
– Be cautious when working with navigation properties in EF. Ensure that you use eager loading or explicit loading to fetch related data efficiently and avoid N+1 query issues.
- Caching:
– Consider caching frequently used data in memory to reduce database round trips. This is particularly helpful for data that doesn’t change frequently.
- Database Tuning:
– Monitor and optimize your database server’s performance. Ensure that it’s properly configured, and regularly analyze query execution plans to identify bottlenecks.
- Use Compiled Queries:
– Entity Framework allows you to compile LINQ queries, which can improve query execution speed by reusing the query execution plan.
- Avoid SELECT * Queries:
– Specify the exact columns you need in your queries instead of using `SELECT *`. Fetching unnecessary columns can increase query execution time and data transfer.
- Logging and Profiling:
– Enable EF logging and profiling tools to track the generated SQL queries. This helps you identify inefficient queries and optimize them.
- Database Index Maintenance:
– Regularly maintain your database indexes by rebuilding or reorganizing them based on fragmentation levels.
- Use AsNoTracking:
– When you don’t intend to modify retrieved entities, use the `AsNoTracking` method to inform EF that it doesn’t need to track changes. This can improve query performance.
By implementing these optimization techniques and continuously monitoring your application’s performance, you can ensure that your Entity Framework-based application runs efficiently and delivers a responsive user experience.
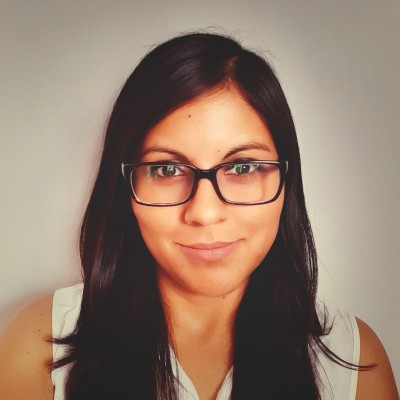
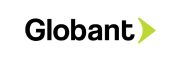