C# Q & A
What is Razor Pages in C#?
Razor Pages is a feature in ASP.NET Core, which is a modern and open-source web framework for building web applications in C#. It provides a simple and lightweight way to create web pages with server-side code and is particularly well-suited for building small to medium-sized web applications. Here are some key points about Razor Pages in C#:
- Page-Oriented Model: Razor Pages follow a page-oriented model, where each web page is represented by a Razor Page file with a `.cshtml` extension. These pages are typically organized within a folder structure.
- Code-Behind: Each Razor Page can have a code-behind file (a `.cshtml.cs` file) that contains the C# code for handling requests, managing data, and defining the page’s behavior. This separation of concerns allows for clean and maintainable code.
- Model Binding: Razor Pages support model binding, which means you can easily bind data from HTTP requests (e.g., form submissions) to C# model classes, making it convenient to work with incoming data.
- Routing: Routing in Razor Pages is based on conventions, where the URL structure directly maps to the folder and file structure of your pages. This results in clean and SEO-friendly URLs.
- View Component: Razor Pages provide a built-in way to create reusable UI components called View Components. These components can be used to encapsulate complex UI logic and rendering.
- Integrated with ASP.NET Core: Razor Pages seamlessly integrate with ASP.NET Core, making use of its features like dependency injection, middleware, authentication, and authorization.
- Testability: Razor Pages are designed to be testable, allowing you to write unit tests for your page-specific code. You can mock HTTP requests and responses to verify your page’s behavior.
- Scaffolding: Visual Studio and other development tools provide scaffolding support for Razor Pages, making it easy to generate boilerplate code for CRUD (Create, Read, Update, Delete) operations and forms.
- Razor Syntax: Razor Pages use the Razor syntax for rendering HTML and incorporating C# code within the HTML markup. This syntax is clean, readable, and familiar to C# developers.
- Community and Documentation: ASP.NET Core, including Razor Pages, has a strong and active community with extensive documentation and tutorials available, making it accessible to both beginners and experienced developers.
Razor Pages in C# is an excellent choice for building web applications with a focus on simplicity, maintainability, and rapid development. It strikes a balance between the ease of use of traditional web forms and the flexibility of the MVC (Model-View-Controller) pattern, making it a versatile option for a wide range of web projects.
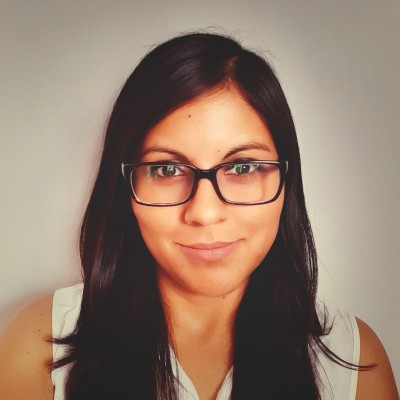
Previously at
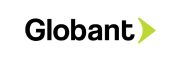
Experienced Backend Developer with 6 years of experience in C#. Proficient in C#, .NET, and Java.Proficient in REST web services and web app development.