How to read and write files in C#?
In C#, reading and writing files is a fundamental task for working with data. You can perform file operations using classes from the `System.IO` namespace. Here’s a concise guide on how to read and write files in C#:
Reading Files:
- Using `File.ReadAllText()`:
To read the entire contents of a text file into a string, you can use the `File.ReadAllText()` method. For example:
```csharp using System.IO; string content = File.ReadAllText("example.txt"); ```
- Using `StreamReader`:
For more control over reading and processing lines from a text file, you can use a `StreamReader`:
```csharp using System.IO; using (StreamReader reader = new StreamReader("example.txt")) { string line; while ((line = reader.ReadLine()) != null) { // Process each line Console.WriteLine(line); } } ```
Writing Files:
- Using `File.WriteAllText()`:
To write a string to a text file, you can use the `File.WriteAllText()` method:
```csharp using System.IO; string content = "Hello, World!"; File.WriteAllText("output.txt", content); ```
- Using `StreamWriter`:
For more advanced writing scenarios, such as appending to an existing file or specifying encoding, you can use a `StreamWriter`:
```csharp using System.IO; using (StreamWriter writer = new StreamWriter("output.txt", true)) { writer.WriteLine("Appended line."); } ```
- Binary File Writing:
When working with binary files, like images or videos, you can use the `FileStream` class to read and write binary data.
Remember to handle exceptions and use try-catch blocks when dealing with file operations to ensure proper error handling, especially when files may not exist or permissions are insufficient. Properly closing file streams and disposing of objects is also crucial to prevent resource leaks.
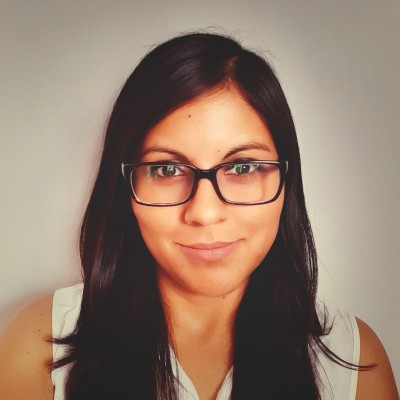
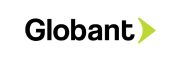