C# Q & A
How to create RESTful endpoints in ASP.NET Core MVC?
Creating RESTful endpoints in ASP.NET Core MVC is a fundamental aspect of building web applications that adhere to the principles of Representational State Transfer (REST). RESTful endpoints allow clients to interact with your application’s resources using standard HTTP methods (GET, POST, PUT, DELETE) and URLs. Here’s how you can create RESTful endpoints in ASP.NET Core MVC:
- Set Up Your ASP.NET Core Project: Begin by creating or opening an ASP.NET Core MVC project in your preferred development environment, such as Visual Studio or Visual Studio Code.
- Define a Model: Identify the resource you want to expose via RESTful endpoints and create a corresponding C# model class to represent it. This class should define the structure of your data, including properties, validation rules, and any business logic related to the resource.
- Create a Controller: In ASP.NET Core MVC, controllers are responsible for handling HTTP requests. Create a controller for your resource by adding a new controller class or scaffolding it using the built-in tools. Your controller should inherit from `ControllerBase` and define actions to handle various HTTP methods.
- Define RESTful Routes: Define routes for your RESTful endpoints using attribute routing or conventional routing. Attribute routing involves decorating controller actions with attributes like `[HttpGet]`, `[HttpPost]`, `[HttpPut]`, and `[HttpDelete]` to specify the supported HTTP methods and URL patterns.
- Implement CRUD Operations: In your controller actions, implement Create (POST), Read (GET), Update (PUT), and Delete (DELETE) operations based on the RESTful principles. Use the model to validate and manipulate data.
- Return Appropriate Responses: Ensure that your controller actions return appropriate HTTP status codes and responses. For example, return HTTP 201 (Created) for successful resource creation and HTTP 200 (OK) for successful retrievals. Use `ActionResult` types to encapsulate your responses.
- Use Input Models and DTOs: To ensure data integrity and security, use input models and Data Transfer Objects (DTOs) to map incoming requests and outgoing responses. This helps in validating and sanitizing data and controlling what is exposed to the client.
- Test Your Endpoints: Test your RESTful endpoints using tools like Postman or by writing unit tests. Verify that your endpoints behave as expected, handle errors gracefully, and return meaningful responses.
- Implement Authorization and Authentication: Depending on your application’s requirements, implement authentication and authorization mechanisms to secure your RESTful endpoints and protect sensitive data.
- Document Your API: Consider documenting your API using tools like Swagger or writing comprehensive API documentation to assist consumers of your API in understanding its endpoints, request/response formats, and usage.
By following these steps, you can create RESTful endpoints in ASP.NET Core MVC that adhere to REST principles, making your application more scalable, maintainable, and interoperable with other systems.
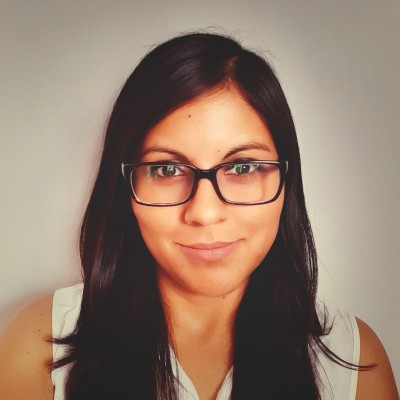
Previously at
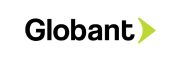
Experienced Backend Developer with 6 years of experience in C#. Proficient in C#, .NET, and Java.Proficient in REST web services and web app development.