How to implement a singleton pattern in C#?
Implementing a singleton pattern in C# ensures that a class has only one instance and provides a global point of access to that instance. This can be useful when you want to control access to a shared resource, ensure a single point of configuration, or maintain a single instance of a class for efficiency reasons. Here’s a common way to implement the singleton pattern in C#:
- Private Constructor: To prevent external instantiation of the class, make the class’s constructor private. This ensures that no other instances of the class can be created from outside the class.
- Private Static Instance: Declare a private static field to hold the single instance of the class within the class itself. Initialize it to null.
- Public Static Method: Create a public static method, often named `GetInstance()` or similar, that provides access to the single instance of the class. Inside this method, check if the instance is null. If it is, create a new instance; otherwise, return the existing instance.
- Thread Safety (Optional): If your application may run in a multi-threaded environment, consider adding synchronization mechanisms like a lock to ensure that only one thread creates the instance when necessary. This helps prevent race conditions during initialization.
Here’s a simplified example of a singleton pattern implementation in C#:
```csharp public class Singleton { private static Singleton instance; // Private constructor to prevent external instantiation private Singleton() { } // Public static method to access the single instance public static Singleton GetInstance() { if (instance == null) { instance = new Singleton(); } return instance; } } ```
With this implementation, you can ensure that only one instance of the `Singleton` class exists throughout the lifetime of your application, and it can be accessed via the `GetInstance()` method. This pattern is useful for scenarios where you need centralized control or coordination, such as managing configuration settings, database connections, or resource pools.
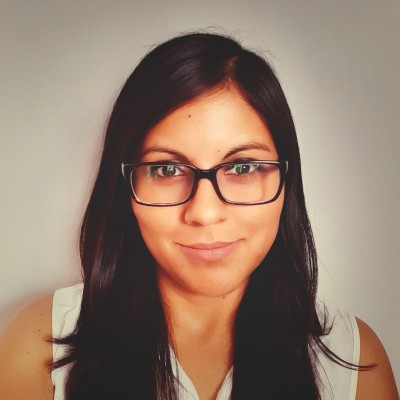
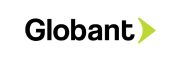