What is the using statement in C#?
In C#, the `using` statement is used primarily for two distinct purposes, each related to resource management, and it helps ensure that resources are properly handled and released. Here’s what the `using` statement does:
- Automatic Resource Management:
The primary use of the `using` statement is to automatically manage resources that implement the `IDisposable` interface. These resources include file streams, database connections, network sockets, and more. When you wrap a resource allocation within a `using` block, the resource is automatically disposed of when the block’s execution scope is exited. This ensures that the resource is released promptly and prevents resource leaks. Here’s an example:
```csharp using (var fileStream = new FileStream("example.txt", FileMode.Open)) { // Work with the fileStream } // When this block is exited, the fileStream is automatically disposed of. ```
- Namespace Importing:
The `using` statement is also used to import namespaces, making types within those namespaces available without requiring fully qualified type names. This simplifies code by allowing you to reference types directly. For instance:
```csharp using System; // Import the System namespace class Program { static void Main() { Console.WriteLine("Hello, World!"); // You can use Console directly. } } ```
Without the `using System` directive, you would need to use `System.Console.WriteLine(“Hello, World!”);` to access the `Console` class.
The `using` statement in C# serves a dual purpose: automatic resource management for `IDisposable` resources and simplifying the referencing of types within namespaces. By using the `using` statement, you can write cleaner, more readable, and resource-safe code in your C# applications.
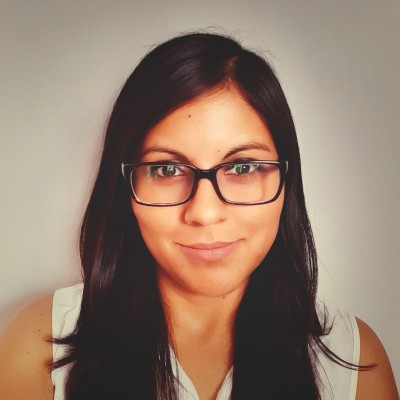
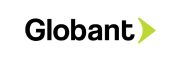