Building and Deploying Web Applications with C# and ASP.NET
When it comes to web application development, C# and ASP.NET represent a powerful duo that is favored by many developers worldwide, making it essential to hire C# developers with proficiency in these areas. C# is an expressive, modern, object-oriented language, while ASP.NET is a robust open-source server-side web application framework, both created by Microsoft. These tools offer a flexible and efficient approach to building everything from simple websites to complex web applications.
In this blog post, we’re going to see how to use these technologies in tandem, and why it’s beneficial to hire C# developers familiar with them, complete with examples.
Background: C# and ASP.NET
C# (“C sharp”) is a statically-typed, object-oriented programming language developed by Microsoft. It combines the power and flexibility of C++ with the simplicity of Visual Basic. The language is highly versatile and can be used to develop a wide range of applications, including desktop applications, mobile apps, web applications, and even game development through platforms like Unity.
ASP.NET is a framework designed to build dynamic web pages and web services using .NET and C#. It uses a server-side model to deliver dynamic web pages, performing processing on the server before sending the webpage to the user’s browser. This allows for advanced interactions, functionalities, as well as improved performance and security.
Setting up the Development Environment
Before embarking on our journey, let’s ensure our environment is set up correctly. This process is something that any organization looking to hire C# developers should be familiar with. Install the .NET Core SDK, which includes everything needed to develop with C# and ASP.NET. Next, download Visual Studio, a feature-packed integrated development environment (IDE) from Microsoft. This IDE is widely used by industry professionals, so when you hire C# developers, they’ll likely be familiar with it. Visual Studio provides an array of tools to improve your development experience and efficiency.
Building a Basic Web Application
Let’s start with creating a simple web application using C# and ASP.NET. Follow along with these steps:
- Creating a new project: Open Visual Studio and create a new project. Choose the “ASP.NET Core Web Application” template, provide a name and location for your project, then click “Create.”
- Configuring the project: Choose the “Web Application (Model-View-Controller)” option and make sure the authentication is set to “No Authentication”. Click “Create” again.
- Understanding the project structure: A new project includes several files and folders. Key directories include:
– ‘Controllers’: Contains classes that handle user input.
– ‘Views’: Holds files responsible for rendering the user interface.
– ‘Models’: Houses classes that represent the application’s data.
– ‘wwwroot’: A web root directory for static files like CSS and JavaScript.
- Creating a Controller: Right-click the “Controllers” folder, then select “Add” > “Controller”. Choose “MVC Controller – Empty”, name it “HomeController”, and click “Add”. This will create a new controller with an Index method, which will be called when a user navigates to your application’s homepage.
- Creating a View: Navigate to the ‘Views’ folder and create a new folder named ‘Home’. Inside it, create a new View called ‘Index.cshtml’. Here, you can use HTML and Razor syntax to design your homepage. Razor syntax is a server-side markup language that allows C# code to be included in HTML files.
```html @{ ViewData["Title"] = "Home Page"; } <div class="text-center"> <h1 class="display-4">Welcome</h1> <p>This is your new homepage.</p> </div>
- Running the application: Press “Ctrl + F5” to run your application. The application will start, and a new browser window will open displaying your newly designed homepage.
Expanding Functionality: Adding a Contact Form
To make our application a bit more interactive let’s add a contact form. Here are the steps to achieve this:
1. Creating a Model: In the ‘Models’ folder, create a new class ‘ContactModel.cs’. This class will represent the data of our contact form.
```csharp public class ContactModel { public string Name { get; set; } public string Email { get; set; } public string Message { get; set; } }
2. Updating the Controller: In ‘HomeController.cs’, add a new action ‘Contact’ that will display the contact form.
```csharp public IActionResult Contact() { return View(); }
3. Creating a View: In the ‘Views/Home’ folder, add a new View named ‘Contact.cshtml’. This View will contain our contact form.
```html @model ContactModel <form asp-action="Submit"> <div> <label asp-for="Name"></label> <input asp-for="Name" /> </div> <div> <label asp-for="Email"></label> <input asp-for="Email" /> </div> <div> <label asp-for="Message"></label> <textarea asp-for="Message"></textarea> </div> <button type="submit">Submit</button> </form>
4. Handling Form Submission: Finally, add another action to ‘HomeController.cs’ that will handle form submission.
```csharp [HttpPost] public IActionResult Submit(ContactModel model) { // Handle form submission here return RedirectToAction("Index"); }
Deployment
Once your web application meets your satisfaction, the next step is to deploy it. You can deploy ASP.NET applications on numerous platforms including Windows, Linux, and macOS. One common option is to deploy to Azure, Microsoft’s cloud platform. Azure offers a plethora of services to support your application such as database services, storage services, and scaling options to manage varying traffic loads.
Conclusion
This blog post explored the essentials of web application development using C# and ASP.NET, offering a glimpse into the skills you’d want when you hire C# developers. We walked through setting up the development environment, creating a basic web application, adding a contact form, and finally discussing deployment.
This is just the tip of the iceberg. C# and ASP.NET offer vast opportunities for creating versatile web applications, an expertise that stands out when you’re looking to hire C# developers. From here, I encourage you to experiment, build, and learn. Resources are plentiful online, including Microsoft’s official documentation, online tutorials, and community forums. These tools, coupled with the right talent you hire as C# developers, can open up unlimited possibilities with C# and ASP.NET. Happy coding!
Table of Contents
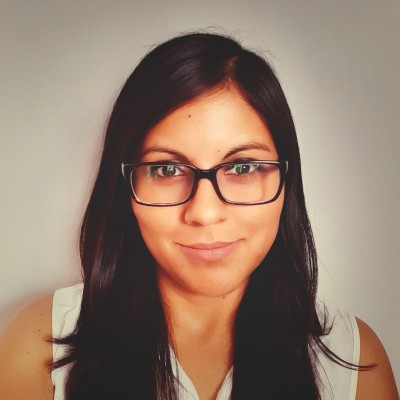
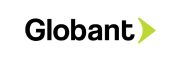