CodeIgniter Meets AJAX: Tips, Tricks, and Best Practices for Web Developers
CodeIgniter, a powerful PHP framework, has been a favorite of many developers for building robust web applications. For those who aim for perfection, many choose to hire CodeIgniter developers to ensure top-notch integration. When combined with AJAX, CodeIgniter can provide a seamless user experience, eliminating the need for page reloads for many actions. Let’s explore how you can incorporate AJAX into your CodeIgniter project and create more dynamic and interactive web applications.
Table of Contents
1. Why Use AJAX in CodeIgniter?
AJAX (Asynchronous JavaScript And XML) lets the web page communicate with the server without having to reload the page. This results in:
– Faster interactions: Instant feedback to users.
– Reduced server load: Since only required data is exchanged.
– Enhanced user experience: Seamless transitions and instantaneous updates.
2. Setting Up CodeIgniter
Before delving into AJAX, ensure you have CodeIgniter set up. Download the latest version from the official site, extract it, and configure `application/config/config.php` and `application/config/database.php` as per your needs.
3. A Simple AJAX Example: Fetching User Details
Imagine we have a system where we want to fetch details of a user without reloading the page. We’ll have an input where we enter the user’s ID, and upon clicking a button, user details will be shown.
3.1. HTML View (`application/views/user_view.php`):
```html <input type="text" id="user_id" placeholder="Enter User ID"> <button id="fetchUser">Fetch User Details</button> <div id="userDetails"></div> <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> <script> $(document).ready(function() { $('#fetchUser').click(function() { var user_id = $('#user_id').val(); $.ajax({ url: "<?php echo base_url('user/fetch');?>", method: "POST", data: {id: user_id}, dataType: "json", success: function(data) { $('#userDetails').html('<p>Name: ' + data.name + '</p><p>Email: ' + data.email + '</p>'); } }); }); }); </script> ```
3.2. CodeIgniter Controller (`application/controllers/User.php`):
```php <?php class User extends CI_Controller { public function __construct() { parent::__construct(); $this->load->model('user_model'); } public function fetch() { $user_id = $this->input->post('id'); $data = $this->user_model->get_user_by_id($user_id); echo json_encode($data); } } ?> ```
3.3. CodeIgniter Model (`application/models/User_model.php`):
```php <?php class User_model extends CI_Model { public function get_user_by_id($id) { $this->db->where('id', $id); $query = $this->db->get('users'); return $query->row_array(); } } ?> ```
In the example above, we’re making an AJAX call to the `fetch` method of the `User` controller, which fetches the user’s data from the database and returns it in JSON format.
4. Handling AJAX Errors
It’s important to handle errors gracefully. With AJAX, this can be achieved using the `error` callback:
```javascript $.ajax({ // ... other options error: function(xhr, status, error) { alert("An error occurred: " + status + " " + error); } }); ```
5. Submitting Forms with AJAX
Instead of simple data fetching, you can also submit entire forms with AJAX. This is especially useful for login forms, comment submissions, and more.
HTML View:
```html <form id="commentForm"> <input type="text" name="comment" placeholder="Your comment"> <button type="submit">Post Comment</button> </form> <script> $('#commentForm').submit(function(e) { e.preventDefault(); $.ajax({ url: "<?php echo base_url('comments/post');?>", method: "POST", data: $(this).serialize(), success: function(data) { alert("Comment posted successfully!"); } }); }); </script> ```
Conclusion
Merging the power of CodeIgniter and AJAX can elevate your web applications to a new level of interactivity. If you’re looking to harness this power, consider the expertise of hire CodeIgniter developers. As you can see, integrating AJAX with CodeIgniter is straightforward. By mastering this combination, you can build web applications that offer faster feedback, improved user engagement, and reduced server load. The key lies in understanding the asynchronous nature of AJAX and designing your CodeIgniter application to handle such requests efficiently. Happy coding!
Table of Contents
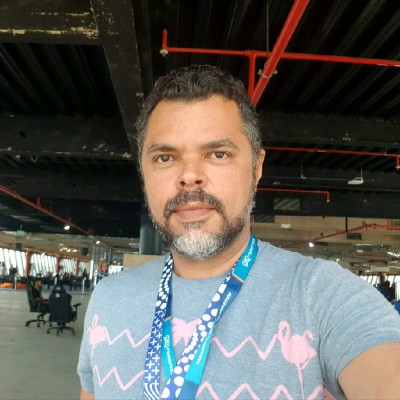
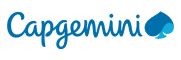