Crafting Modern Web Apps: A Deep Dive into CodeIgniter and AngularJS Integration
Web development is a constantly evolving domain, and developers often find themselves juggling with multiple tools to create cutting-edge web apps. With businesses frequently looking to hire CodeIgniter developers for backend expertise, it’s evident that CodeIgniter, a PHP MVC (Model-View-Controller) framework, holds a significant place in this arena.
Table of Contents
On the other hand, AngularJS, a robust JavaScript framework maintained by Google, completes the equation. When combined, these frameworks can help developers build highly interactive and data-driven web applications. This article delves into creating a dynamic web app using these two technologies, emphasizing the importance and value when you hire CodeIgniter developers.
1. Why CodeIgniter and AngularJS?
– CodeIgniter: Known for its lightweight, straightforward setup and exceptional performance, CodeIgniter is a favorite among PHP developers. Its MVC architecture allows for separation of logic and presentation, making code management efficient.
– AngularJS: A front-end framework that excels in creating single-page applications (SPAs). It brings two-way data binding, dependency injection, and a modular architecture, resulting in highly interactive web interfaces.
2. Setting up CodeIgniter and AngularJS
2.1. CodeIgniter:
- Download the latest version from the [official website] (https://codeigniter.com/).
- Extract the zip file and place the contents in your web server directory.
2.2. AngularJS:
- Include AngularJS via CDN or download from the [AngularJS website] (https://angularjs.org/).
- Link it in your HTML file:
```html <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script> ```
3. Developing a Simple CRUD App
For this example, let’s create a task manager where users can add, view, update, and delete tasks.
3.1. Backend with CodeIgniter:
Model – Create a file `Task_model.php` inside `application/models/`.
```php class Task_model extends CI_Model { public function get_tasks() { $query = $this->db->get('tasks'); return $query->result(); } // ... add methods for create, update, delete. } ```
Controller – Create a file `Task.php` inside `application/controllers/`.
```php class Task extends CI_Controller { public function get_all_tasks() { $this->load->model('Task_model'); $tasks = $this->Task_model->get_tasks(); echo json_encode($tasks); } // ... add methods for create, update, delete. } ```
3.2. Frontend with AngularJS:
Create an AngularJS controller, `TaskController.js`.
```javascript var app = angular.module('taskApp', []); app.controller('TaskController', function($scope, $http) { $scope.tasks = []; $scope.getAllTasks = function() { $http.get('/index.php/Task/get_all_tasks').then(function(response) { $scope.tasks = response.data; }); }; // ... add methods for create, update, delete. $scope.getAllTasks(); // Get tasks on page load. }); ```
In your HTML:
```html <!DOCTYPE html> <html ng-app="taskApp"> <head> <!-- ... other headers ... --> <script src="TaskController.js"></script> </head> <body ng-controller="TaskController"> <ul> <li ng-repeat="task in tasks">{{ task.name }}</li> </ul> <!-- ... CRUD functionalities ... --> </body> </html> ```
4. Advantages of this Duo
- Scalability: CodeIgniter’s lightweight nature combined with AngularJS’s modular architecture makes scaling applications effortless.
- Speed: CodeIgniter is known for its performance, and AngularJS reduces server load by handling much of the processing on the client-side.
- Reusability: AngularJS allows developers to create custom directives, promoting code reuse, while CodeIgniter’s MVC architecture separates concerns efficiently.
5. Considerations
– Learning Curve: Both frameworks have their idiosyncrasies. However, the vibrant communities around both make it easier.
– Version Maintenance: Ensure compatibility between versions of both frameworks.
Conclusion
The union of CodeIgniter and AngularJS offers a powerful platform for developing dynamic web applications. As businesses increasingly hire CodeIgniter developers for backend proficiency, it’s evident that CodeIgniter handles the server-side processes with finesse. In tandem, AngularJS provides interactivity and real-time response on the client side. When combined with best practices, this duo can lead to the creation of web applications that are efficient, maintainable, and highly interactive.
Remember, the example above is a simplified demonstration of what can be achieved when you harness the expertise of skilled CodeIgniter developers. Dive deep, and you’ll uncover the true potential of these tools.
Table of Contents
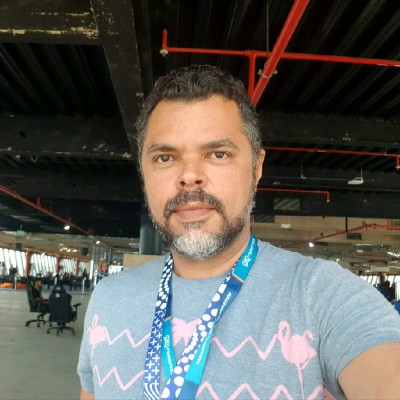
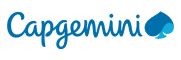