CodeIgniter and Augmented Reality: Building AR-Enabled Applications
Augmented Reality (AR) is transforming the way we interact with digital content by overlaying virtual elements onto the real world. For web developers, integrating AR into applications can enhance user experience and engagement. In this blog, we’ll explore how to build AR-enabled applications using the CodeIgniter framework.
Getting Started with CodeIgniter and AR
CodeIgniter is a powerful PHP framework known for its speed and small footprint. It is a popular choice for building dynamic web applications. When combined with AR technology, it can create immersive experiences for users. Let’s dive into the initial setup.
Setting Up the Environment
To start, ensure you have the latest version of CodeIgniter installed. Follow these steps:
Download CodeIgniter:
```sh composer create-project codeigniter4/appstarter codeigniter-ar ```
- Set Up Your Web Server: Configure your local or remote server to serve the CodeIgniter project. You can use Apache, Nginx, or any other server of your choice.
- Install Dependencies: AR requires client-side technologies like JavaScript libraries and frameworks. We will use AR.js, a lightweight library for creating AR experiences on the web.
Integrating AR.js with CodeIgniter
Now that CodeIgniter is set up, let’s integrate AR.js. This JavaScript library allows us to add AR features using technologies like WebGL and WebRTC.
Include AR.js in Your View:
Create a new view file in app/Views called ar_view.php and include AR.js:
```html <!DOCTYPE html> <html> <head> <title>AR Example</title> <script src="https://aframe.io/releases/1.0.4/aframe.min.js"></script> <script src="https://raw.githack.com/AR-js-org/AR.js/3.3.2/aframe/build/aframe-ar.js"></script> </head> <body> <a-scene embedded arjs> <a-box position='0 0.5 0' material='opacity: 0.5;'></a-box> <a-marker-camera preset='hiro'></a-marker-camera> </a-scene> </body> </html> ```
Create a Controller to Serve the View:
In app/Controllers, create a controller called ARController.php:
```php <?php namespace App\Controllers; use CodeIgniter\Controller; class ARController extends Controller { public function index() { return view('ar_view'); } } ```
Configure Routes:
Update the routing configuration in app/Config/Routes.php:
```php $routes->get('/ar', 'ARController::index'); ```
Developing AR Features
With the basic setup complete, you can start adding more complex AR elements. AR.js supports various features such as image recognition, marker tracking, and geolocation-based AR.
Adding Image Recognition
To implement image recognition, you need to:
- Prepare an Image Marker: Create or download a marker image. This image will trigger the AR experience.
Modify the View:
Update the ar_view.php file to include image recognition:
```html <a-marker type='pattern' url='path/to/pattern-marker.patt'> <a-box position='0 0.5 0' material='color: red;'></a-box> </a-marker> ```
2.
Handling Interactions:
You can add interactions using JavaScript. For example, changing the color of a box when it is clicked:
```html <a-box id='box' position='0 0.5 0' material='color: red;' onclick="this.setAttribute('material', 'color: blue');"></a-box> ```
3.
Conclusion
Building AR-enabled applications with CodeIgniter is a fascinating journey that combines the power of a robust PHP framework with cutting-edge AR technology. By leveraging libraries like AR.js, you can create immersive experiences that engage users in new and exciting ways.
Further Reading
Table of Contents
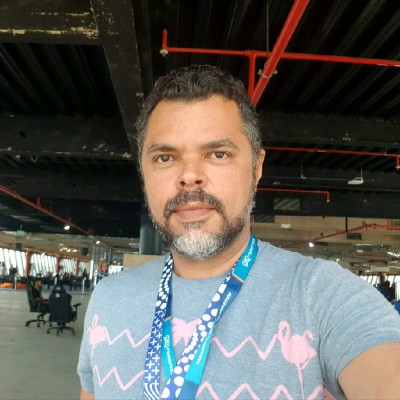
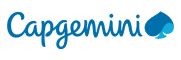