Seamless Sign-ins: OAuth’s Role in CodeIgniter Authentication
The digital world we live in today demands seamless user experiences. A significant part of this user experience is the authentication process. Users have countless accounts and passwords to remember. A method to simplify this process is using OAuth for authentication.
1. What is OAuth?
OAuth (Open Authorization) is an open standard for token-based authentication and authorization. In simpler terms, it allows third-party services to exchange your information without you having to give away your password. For instance, when you log into a website using your Google or Facebook account, you’re using OAuth.
2. Why integrate OAuth with CodeIgniter?
CodeIgniter, a robust PHP framework, offers a lightweight, performance-optimized platform for web application development. By integrating OAuth with CodeIgniter, developers can provide users with a quick, efficient, and secure login process.
3. Steps to Integrate OAuth with CodeIgniter
- Set up CodeIgniter: Ensure you have the latest version of CodeIgniter installed and properly configured.
- Choose an OAuth library: There are multiple OAuth libraries available for CodeIgniter, like OAuth2 or HybridAuth. For this post, we’ll use `HybridAuth`.
- Install HybridAuth: Download and integrate the HybridAuth library into your CodeIgniter installation. Typically, you would place it in the third_party folder of your application directory.
- Configuration: Configure your selected providers (e.g., Google, Facebook). Open the configuration file (typically `hybridauthlib.php`), and insert your application credentials provided by the OAuth service providers.
```php $config['providers'] = array( 'Google' => array( 'enabled' => TRUE, 'keys' => array('id' => 'YOUR_GOOGLE_ID', 'secret' => 'YOUR_GOOGLE_SECRET'), ), 'Facebook' => array( 'enabled' => TRUE, 'keys' => array('id' => 'YOUR_FACEBOOK_ID', 'secret' => 'YOUR_FACEBOOK_SECRET'), ) ); ```
- User Login: Create a method in your controller to handle user login. This method redirects the user to the chosen OAuth provider.
```php public function login($provider) { try { $this->load->library('hybridauthlib'); $service = $this->hybridauthlib->authenticate($provider); if ($service->isUserConnected()) { $user_profile = $service->getUserProfile(); // Store $user_profile details into the session or database. } } catch (Exception $e) { show_error($e->getMessage()); } } ```
This `login` method initiates the authentication process for the selected provider.
- User Logout:
It’s equally crucial to handle user logouts efficiently. For that:
```php public function logout($provider) { try { $this->load->library('hybridauthlib'); $service = $this->hybridauthlib->getAdapter($provider); $service->logout(); } catch (Exception $e) { show_error($e->getMessage()); } } ```
- Front-end Implementation:
Now, on your login page, you can create buttons or links to direct users to the OAuth providers:
```html <a href="<?= base_url() ?>yourcontroller/login/google">Login with Google</a> <a href="<?= base_url() ?>yourcontroller/login/facebook">Login with Facebook</a> ```
4. Advantages of using OAuth with CodeIgniter
– Enhanced Security: OAuth reduces the risks associated with the mishandling of user passwords. It uses access tokens rather than user credentials.
– Reduced Friction: Users can log in faster since they don’t need to fill in registration forms or remember another password.
– Increased Trust: Many users trust big names like Google or Facebook and feel more comfortable logging in with their accounts.
Conclusion
OAuth authentication with CodeIgniter simplifies the login process and enhances user experience. With libraries like HybridAuth, integrating OAuth with CodeIgniter becomes a straightforward task. Offering such an authentication mechanism ensures you stay updated with current web trends, providing your users with a trusted and effortless login process.
Table of Contents
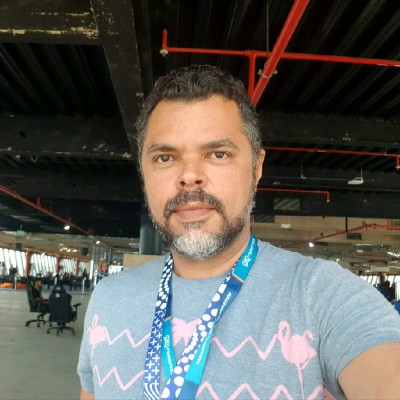
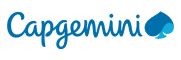