Step-by-Step Guide to Automating Web Tasks with CodeIgniter’s CLI
When it comes to web development, having the ability to automate tasks is paramount for improving efficiency. CodeIgniter, a popular PHP framework, offers a robust Command Line Interface (CLI) tool that can help developers automate a variety of tasks, including the setup of cron jobs. In this article, we will delve into the world of CodeIgniter CLI, provide illustrative examples, and demonstrate how you can automate tasks and set up cron jobs.
Table of Contents
1. What is CodeIgniter CLI?
The CLI in CodeIgniter is a way to interact with the framework using a command line instead of through a web browser. It comes handy for tasks that are more suitable for the command line, such as maintenance tasks, generating boilerplate code, or running scheduled jobs.
2. Setting Up CodeIgniter CLI
Before we dive into the examples, make sure your CodeIgniter setup is CLI-ready:
- Ensure the CLI is accessible: Navigate to your project root and run:
```bash $ php index.php ```
You should see a list of available commands if everything is correctly set up.
- Set your base URL: In `application/config/config.php`, ensure your base URL is set correctly. For CLI requests, it’s advisable to set it explicitly.
```php $config['base_url'] = 'http://your-domain.com/'; ```
3. Automating Tasks with CodeIgniter CLI
Let’s explore how to create and run custom commands using the CLI.
3.1. Creating a Command
To create a command, you can extend the Command class. Let’s say we want a command that greets us:
```php // application/Commands/Greet.php namespace App\Commands; use CodeIgniter\CLI\BaseCommand; use CodeIgniter\CLI\CLI; class Greet extends BaseCommand { protected $group = 'Demo'; protected $name = 'demo:greet'; protected $description = 'Greets the user.'; public function run(array $params) { CLI::write('Hello, CodeIgniter!', 'green'); } } ```
3.2. Running the Command
Navigate to your project root and run:
```bash $ php index.php demo:greet ```
You should see the message “Hello, CodeIgniter!” printed in green.
4. Automating Cron Jobs with CodeIgniter CLI
A common use-case for CLI in web applications is to set up cron jobs – scheduled tasks that run at fixed times or intervals. Here’s how to set up a cron job using CodeIgniter’s CLI:
4.1. Creating a Command for the Cron Job
Suppose you want to clear cache every day at midnight:
```php // application/Commands/ClearCache.php namespace App\Commands; use CodeIgniter\CLI\BaseCommand; use CodeIgniter\CLI\CLI; class ClearCache extends BaseCommand { protected $group = 'Maintenance'; protected $name = 'maint:clearcache'; protected $description = 'Clears the cache.'; public function run(array $params) { // Logic to clear cache // ... CLI::write('Cache cleared!', 'yellow'); } } ```
4.2. Setting Up the Cron Job
On a Linux server, you’d use the `crontab` tool:
- Open the crontab for editing:
```bash $ crontab -e ```
- Add your command to run daily at midnight:
```bash 0 0 * * * /usr/bin/php /path-to-your-project/index.php maint:clearcache ```
This tells the system to execute the `maint:clearcache` command daily at midnight.
5. Caveats and Tips
- Full Paths: Always use full paths in cron jobs to ensure there’s no ambiguity.
- Logging: Redirect the output of the cron job to a log file to capture any messages or errors:
```bash 0 0 * * * /usr/bin/php /path-to-your-project/index.php maint:clearcache >> /path-to-logs/clearcache.log 2>&1 ```
- Environment: Ensure that the PHP being used in the cron job has all the required extensions and is of the correct version.
Conclusion
The CodeIgniter CLI provides a powerful way to automate tasks in your application. By creating custom commands, you can tailor the CLI to fit the unique needs of your project. Furthermore, combining the CLI with cron jobs ensures that your application can conduct routine maintenance, report generation, or any other scheduled task without manual intervention.
Dive into the CodeIgniter documentation and explore the myriad of other functionalities available in the CLI. Harness its power and streamline your development and maintenance processes.
Table of Contents
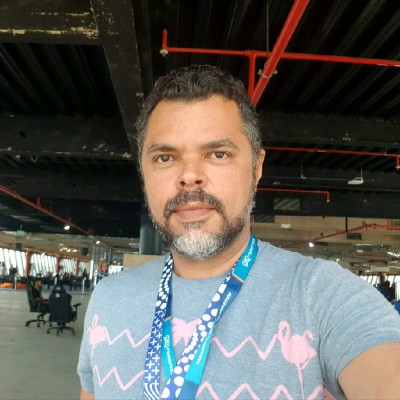
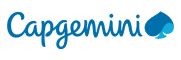