CodeIgniter and Big Data: Processing and Analyzing Massive Data Sets
Big Data has become an integral part of modern data-driven applications. Handling massive data sets efficiently requires robust tools and frameworks. CodeIgniter, a popular PHP framework, is renowned for its simplicity and performance. While not traditionally associated with Big Data, it can be effectively used to manage and process large volumes of data when combined with the right strategies and tools. In this blog, we’ll explore how CodeIgniter can be utilized in the context of Big Data, covering key aspects of data processing and analysis.
Understanding Big Data and Its Challenges
Big Data refers to extremely large data sets that may be structured, semi-structured, or unstructured. These data sets are often characterized by the 3 Vs: Volume, Velocity, and Variety. Processing and analyzing Big Data involves several challenges:
- Volume: Managing and storing large amounts of data.
- Velocity: Handling the speed at which data is generated and needs to be processed.
- Variety: Integrating and analyzing different types of data.
Integrating CodeIgniter with Big Data Technologies
Although CodeIgniter itself does not provide native support for Big Data technologies, it can be integrated with various tools and platforms to handle large-scale data processing. Here are a few approaches to achieve this integration:
1. Using CodeIgniter with Apache Hadoop
Apache Hadoop is a popular framework for processing and storing large data sets across distributed systems. To integrate CodeIgniter with Hadoop, you can use PHP libraries that interface with Hadoop’s APIs. For example, the php-hadoop library allows PHP applications to interact with Hadoop.
Example Code: Connecting to Hadoop
```php <?php require 'vendor/autoload.php'; use Hadoop\HadoopClient; $client = new HadoopClient([ 'host' => 'hadoop-server', 'port' => '9000' ]); $response = $client->get('/path/to/hadoop/file'); echo $response; ?> ```
2. Leveraging CodeIgniter with Apache Spark
Apache Spark is another powerful tool for Big Data processing. Spark provides an API for PHP, which can be used alongside CodeIgniter to perform complex data analysis tasks. For PHP applications, you might need to use a REST API to interact with Spark.
Example Code: Interacting with Spark REST API
```php <?php $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, "http://spark-server:4040/api/v1/applications"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); $response = curl_exec($ch); curl_close($ch); $data = json_decode($response, true); print_r($data); ?> ```
3. Implementing Data Storage Solutions
For managing large volumes of data, integrating CodeIgniter with a scalable database solution is crucial. Consider using NoSQL databases like MongoDB or Cassandra for handling large datasets. CodeIgniter’s database library can be extended to interact with these databases.
Example Code: Using MongoDB with CodeIgniter
```php <?php $mongo = new MongoDB\Client("mongodb://localhost:27017"); $collection = $mongo->mydatabase->mycollection; $result = $collection->find([]); foreach ($result as $entry) { echo $entry['field'] . "<br>"; } ?> ```
Best Practices for Big Data with CodeIgniter
- Optimize Database Queries: Use indexing and efficient query design to improve performance when dealing with large data sets.
- Asynchronous Processing: Offload time-consuming tasks to background processes or queues to ensure that your application remains responsive.
- Caching: Implement caching strategies to reduce the load on your database and speed up data retrieval.
Conclusion
While CodeIgniter is not a Big Data framework per se, it can be effectively used in conjunction with other Big Data technologies to build powerful data-driven applications. By integrating CodeIgniter with tools like Hadoop and Spark, and optimizing data handling strategies, developers can leverage its simplicity and performance in Big Data scenarios.
Further Reading
Table of Contents
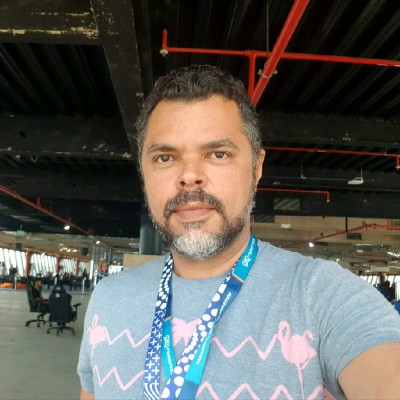
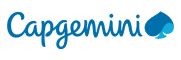