Blade vs. Twig: Enhancing Your CodeIgniter Web Development Experience
CodeIgniter is a powerful PHP framework that offers a lightweight and straightforward platform to develop web applications. Recognizing its potential, many choose to hire CodeIgniter developers for optimized solutions. One of its standout features is its easy extensibility. While CodeIgniter does have a built-in mechanism for managing views, some developers have a penchant for the more advanced templating engines, like Blade (from the Laravel framework) or Twig. These engines step up the game with enhanced features such as template inheritance and a much cleaner syntax.
Table of Contents
In this post, we’ll navigate you through the intricacies of integrating either Blade or Twig with CodeIgniter.
1. Why Use a Templating Engine?
Before diving into the integration, it’s essential to understand why you’d want to use a templating engine in the first place:
- Simplified Syntax: Templating engines offer a cleaner syntax for rendering dynamic data, making it easier to read and maintain.
- Template Inheritance: This feature allows you to define a base template and extend it in other templates, ensuring consistency and reducing code duplication.
- Security: Engines like Twig automatically escape output, reducing the risk of XSS attacks.
- Extensibility: You can easily add custom functions, filters, and extensions.
2. Integrating Blade with CodeIgniter:
2.1. Setup:
Firstly, you’ll need to install Blade. One way to do this is through Composer:
```bash composer require illuminate/view ```
2.2. Configuration:
- Service Provider: Create a service provider for Blade:
```php // application/providers/BladeServiceProvider.php use Illuminate\Container\Container; use Illuminate\Events\Dispatcher; use Illuminate\Filesystem\Filesystem; use Illuminate\View\ViewServiceProvider; class BladeServiceProvider extends ViewServiceProvider { public function register() { $this->app = new Container; $this->app->instance('config', new Collection([ 'view.paths' => [APPPATH . 'views'], 'view.compiled' => APPPATH . 'cache/views', ])); $this->app->singleton('files', function () { return new Filesystem; }); $this->app->singleton('events', function () { return new Dispatcher; }); parent::register(); } } ```
- View Loader: Create a helper function to load views:
```php // application/helpers/blade_helper.php function view($view, $data = []) { $blade = new BladeServiceProvider(new Container); echo $blade->app['view']->make($view, $data); } ```
2.3. Usage:
In your controller:
```php $this->load->helper('blade'); $data = ['name' => 'John']; view('welcome', $data); ```
And your `welcome.blade.php` view file might look something like this:
```php <h1>Hello, {{ $name }}!</h1> ```
3. Integrating Twig with CodeIgniter:
3.1. Setup:
Install Twig via Composer:
```bash composer require twig/twig ```
3.2. Configuration:
- Initialize Twig:
```php // application/libraries/Twig.php use Twig\Environment; use Twig\Loader\FilesystemLoader; class Twig { private $twig; public function __construct() { $loader = new FilesystemLoader(APPPATH . 'views'); $this->twig = new Environment($loader, [ 'cache' => APPPATH . 'cache/views', 'autoescape' => 'html', 'debug' => true, ]); } public function display($view, $data = []) { echo $this->twig->render($view . '.twig', $data); } } ```
3.3. Usage:
In your controller:
```php $this->load->library('twig'); $data = ['name' => 'Jane']; $this->twig->display('welcome', $data); ```
And your `welcome.twig` view might look like:
```twig <h1>Hello, {{ name }}!</h1> ```
Conclusion
By integrating advanced templating engines like Blade or Twig with CodeIgniter, you can leverage the best of both worlds: CodeIgniter’s lightweight framework combined with the powerful templating capabilities of Blade or Twig. If you’re looking to optimize this integration, consider hiring CodeIgniter developers. Choose the engine that best suits your needs and take your CodeIgniter projects to the next level.
Table of Contents
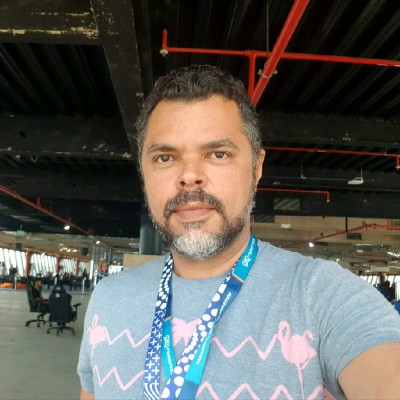
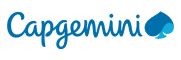