CodeIgniter and Chat Applications: Developing Real-time Chat Systems
In today’s interconnected world, real-time chat applications are becoming increasingly vital for communication. Whether for customer support, social networking, or team collaboration, the demand for seamless and interactive chat solutions is high. CodeIgniter, a popular PHP framework, can be a robust choice for developing such applications. In this blog, we’ll explore how to build a real-time chat system using CodeIgniter, focusing on key components, implementation strategies, and best practices.
Understanding the Basics of Real-time Chat
Real-time chat applications allow users to send and receive messages instantly, without needing to refresh their browsers. To achieve this, chat systems typically rely on technologies such as WebSockets or AJAX polling.
WebSockets vs. AJAX Polling
- WebSockets: This protocol provides full-duplex communication channels over a single TCP connection. It allows for real-time interaction between the client and server, making it ideal for chat applications.
- AJAX Polling: This technique involves repeatedly sending requests to the server to check for new messages. While simpler to implement, it’s less efficient than WebSockets, as it involves more overhead.
Setting Up CodeIgniter for a Chat Application
Before diving into the code, ensure you have CodeIgniter installed and configured on your server. For this tutorial, we’ll use CodeIgniter 4.
Step 1: Create a Chat Database
First, you need a database to store chat messages. Here’s a simple schema for a messages table:
```sql CREATE TABLE `messages` ( `id` INT(11) AUTO_INCREMENT PRIMARY KEY, `user_id` INT(11) NOT NULL, `message` TEXT NOT NULL, `created_at` TIMESTAMP DEFAULT CURRENT_TIMESTAMP ); ```
Step 2: Setting Up the Model
Create a model to interact with the messages table. Save this file as ChatModel.php in the app/Models directory:
```php <?php namespace App\Models; use CodeIgniter\Model; class ChatModel extends Model { protected $table = 'messages'; protected $primaryKey = 'id'; protected $allowedFields = ['user_id', 'message']; protected $useTimestamps = true; public function getMessages() { return $this->orderBy('created_at', 'ASC')->findAll(); } } ```
Step 3: Building the Controller
Create a controller to handle chat logic. Save this file as ChatController.php in the app/Controllers directory:
```php <?php namespace App\Controllers; use App\Models\ChatModel; class ChatController extends BaseController { public function index() { $model = new ChatModel(); $data['messages'] = $model->getMessages(); return view('chat_view', $data); } public function postMessage() { $model = new ChatModel(); $message = $this->request->getPost('message'); $user_id = 1; // Hardcoded for simplicity, replace with dynamic user ID $model->save([ 'user_id' => $user_id, 'message' => $message ]); return redirect()->to('/chat'); } } ```
Step 4: Creating the View
Now, create a view to display and send messages. Save this file as chat_view.php in the app/Views directory:
```html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Chat Application</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function(){ $('#sendButton').click(function(){ var message = $('#messageInput').val(); $.post('/chat/postMessage', {message: message}, function(){ $('#messageInput').val(''); location.reload(); }); }); }); </script> </head> <body> <h1>Chat Application</h1> <div id="chatBox"> <?php foreach ($messages as $message): ?> <p><?php echo $message['message']; ?></p> <?php endforeach; ?> </div> <input type="text" id="messageInput" placeholder="Type a message"> <button id="sendButton">Send</button> </body> </html> ```
Step 5: Adding Real-time Capabilities
To make this chat application real-time, integrate WebSockets. One popular choice is Ratchet, a PHP library for WebSocket communication. Follow Ratchet’s documentation to set up a WebSocket server and connect it with your CodeIgniter application.
Conclusion
Building a real-time chat application with CodeIgniter involves setting up a robust backend with efficient database interactions and implementing real-time communication protocols. By combining CodeIgniter with WebSockets, you can create a responsive and interactive chat system that meets modern communication needs.
Further Reading
Table of Contents
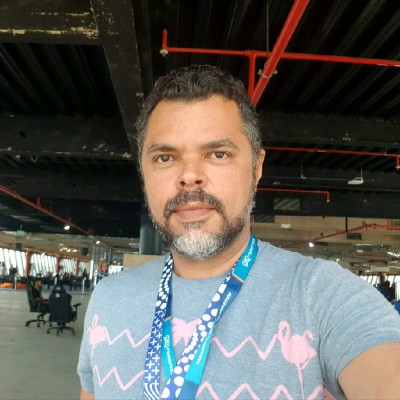
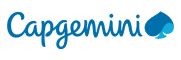