CodeIgniter and Chatbots: Implementing Automated Conversational Interfaces
In the modern digital landscape, chatbots have become essential tools for automating customer interactions, providing instant responses, and enhancing user engagement. Integrating chatbots into web applications is increasingly popular, and frameworks like CodeIgniter make it easier for developers to implement these conversational interfaces efficiently. This blog explores how to build and deploy chatbots in a CodeIgniter environment, providing a step-by-step guide and essential code snippets.
Understanding Chatbots and CodeIgniter
What Are Chatbots?
Chatbots are software applications designed to simulate human-like conversations with users. They can be rule-based, responding to specific inputs, or AI-powered, using machine learning and natural language processing (NLP) to understand and respond to a broader range of inputs.
Why CodeIgniter?
CodeIgniter is a powerful PHP framework known for its simplicity and performance. It provides a structured approach to web development, making it easier to build robust applications. With its MVC architecture, CodeIgniter is ideal for implementing modular chatbot components.
Setting Up Your Development Environment
Prerequisites
Before you start, ensure you have the following:
- A working installation of CodeIgniter
- A database (MySQL or other) for storing conversation logs
- Basic knowledge of PHP and CodeIgniter
Installing Necessary Libraries
To work with chatbots, you may need to install additional libraries or APIs for NLP and machine learning. For simplicity, we’ll use the Dialogflow API, a popular choice for building conversational interfaces.
Example: Installing Dialogflow PHP Client Library
```bash composer require google/cloud-dialogflow ```
Building the Chatbot Interface
Creating the Chatbot Controller
In CodeIgniter, create a new controller named Chatbot.php to handle requests and responses.
```php <?php defined('BASEPATH') OR exit('No direct script access allowed'); class Chatbot extends CI_Controller { public function __construct() { parent::__construct(); // Load necessary models and libraries } public function index() { $this->load->view('chatbot_view'); } public function sendMessage() { $message = $this->input->post('message'); // Logic to send the message to Dialogflow and get a response $response = $this->getResponseFromDialogflow($message); echo json_encode($response); } private function getResponseFromDialogflow($message) { // Implement Dialogflow API call here // Return the response } } ?> ```
Designing the Chatbot View
Create a view file named chatbot_view.php to serve as the chatbot’s user interface.
```html <!DOCTYPE html> <html> <head> <title>Chatbot</title> </head> <body> <div id="chat-container"> <div id="messages"></div> <input type="text" id="message-input" placeholder="Type a message..."> <button id="send-button">Send</button> </div> <script> document.getElementById('send-button').addEventListener('click', function() { var message = document.getElementById('message-input').value; sendMessageToServer(message); }); function sendMessageToServer(message) { // Send the message to the server using AJAX // Display the response in the chat } </script> </body> </html> ```
Integrating Dialogflow API
In the Chatbot controller, implement the getResponseFromDialogflow method to handle API requests.
```php private function getResponseFromDialogflow($message) { // Initialize Dialogflow client $projectId = 'your-project-id'; $sessionId = 'your-session-id'; $languageCode = 'en-US'; $credentials = 'path/to/credentials.json'; $client = new Google\Cloud\Dialogflow\V2\SessionsClient([ 'credentials' => $credentials, ]); $session = $client->sessionName($projectId, $sessionId); $textInput = (new Google\Cloud\Dialogflow\V2\TextInput()) ->setText($message) ->setLanguageCode($languageCode); $queryInput = (new Google\Cloud\Dialogflow\V2\QueryInput()) ->setText($textInput); $response = $client->detectIntent($session, $queryInput); $fulfillmentText = $response->getQueryResult()->getFulfillmentText(); return $fulfillmentText; } ```
Deploying and Testing Your Chatbot
Deployment Considerations
- Security: Ensure secure handling of API keys and sensitive information.
- Scalability: Plan for scaling the chatbot as user interactions grow.
- Monitoring: Implement logging and monitoring for performance and errors.
Testing
Test the chatbot thoroughly to ensure it understands and responds accurately to user inputs. Use various scenarios to test its robustness and adaptability.
Conclusion
Integrating chatbots into CodeIgniter applications enhances user experience by providing instant and automated responses. With the power of CodeIgniter’s framework and APIs like Dialogflow, developers can create sophisticated conversational interfaces that cater to various business needs.
Further Reading
Table of Contents
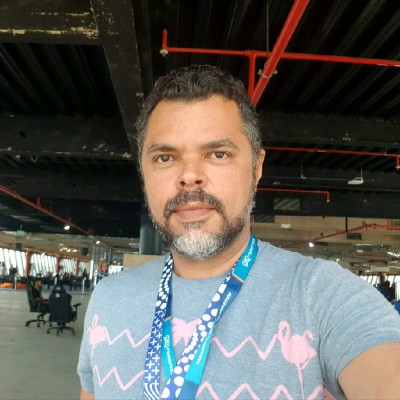
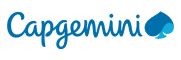