How to create and manage cron jobs in CodeIgniter?
In CodeIgniter, you can create and manage cron jobs to automate recurring tasks by leveraging the built-in support for command-line operations. Here’s a step-by-step guide on how to create and manage cron jobs in CodeIgniter:
- Create a Command-Line Controller:
Start by creating a new controller specifically for handling cron jobs. You can do this using the following command:
``` php spark make:controller Cron ```
This command generates a new controller file named `Cron.php` in your `app/Controllers` directory.
- Define Cron Job Methods:
In the `Cron.php` controller, define methods that correspond to the tasks you want to automate. These methods should contain the logic for your cron jobs. For example:
```php <?php namespace App\Controllers; class Cron extends BaseController { public function nightlyBackup() { // Your backup logic here } public function generateReports() { // Report generation logic here } } ```
- Create a Shell Script:
To execute your CodeIgniter cron jobs from the command line, create a shell script that invokes the appropriate CodeIgniter commands. Here’s an example of a simple shell script:
```bash #!/bin/bash cd /path/to/your/codeigniter/project php spark cron:nightlyBackup php spark cron:generateReports ```
Make sure to replace `/path/to/your/codeigniter/project` with the actual path to your CodeIgniter project.
- Make the Shell Script Executable:
Set the execute permission for your shell script using the following command:
```bash chmod +x /path/to/your/script.sh ```
- Add Cron Jobs:
Use the `crontab` command to add entries for your shell script in the crontab file. Open your crontab for editing:
```bash crontab -e ```
Then, add your cron job entries. For example, to run your script every night at midnight, add the following line:
```bash 0 0 * * * /path/to/your/script.sh ```
- Save and Exit:
Save the changes to your crontab file and exit the editor.
Your CodeIgniter cron jobs are now set up to run at the specified times. Be sure to monitor the output and logs to ensure that your tasks are executing as expected. Additionally, you can add error handling and logging to your CodeIgniter cron job methods to track any issues that may arise during execution.
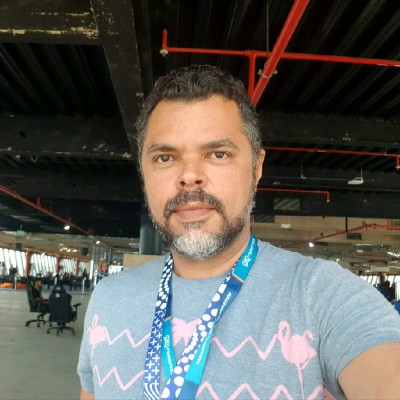
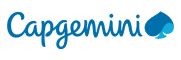