Boost Your CodeIgniter App with Automated Cron Tasks
Cron jobs are scheduled tasks that run automatically at specified intervals. They are especially useful for automating routine tasks, such as sending emails, backing up databases, or generating reports. In this article, we’ll delve into how you can set up cron jobs in a CodeIgniter application, complete with examples.
1. Understanding Cron Syntax
Before diving into CodeIgniter specifics, let’s understand the cron syntax. A typical cron job setting looks like this:
``` * * * * * command-to-be-executed ```
The five asterisks represent:
– Minute (0 – 59)
– Hour (0 – 23)
– Day of the month (1 – 31)
– Month (1 – 12)
– Day of the week (0 – 7) [Both 0 and 7 stand for Sunday]
For example, `0 0 * * *` would mean “run the command every day at midnight.”
2. Setting up a Cron Job in CodeIgniter
With the foundational knowledge of cron syntax, let’s see how to set up a cron job in a CodeIgniter application.
Example: Sending Daily Email Reports
Suppose you have a function in your controller that sends daily email reports:
```php class Reports extends CI_Controller { public function send_daily_report() { // Fetch the data and send email } } ```
To set this function as a cron job:
- Open the terminal and type `crontab -e` to edit the cron file.
- Add the following line at the end of the file:
``` 0 0 * * * wget -q -O - http://yourwebsite.com/index.php/reports/send_daily_report ```
This will ensure that the `send_daily_report` function is called every day at midnight.
3. Using CLI in CodeIgniter
While the above method works, it’s a better practice to use Command Line Interface (CLI) for running cron jobs in CodeIgniter. This way, you can prevent the task from being accidentally triggered by web visitors.
Modify your function to ensure it’s being accessed only from CLI:
```php public function send_daily_report() { if (!$this->input->is_cli_request()) { exit('This script can only be accessed via the command line.'); } // Fetch the data and send email } ```
Then, in your cron file:
``` 0 0 * * * /usr/bin/php /path/to/your/ci/index.php reports send_daily_report ```
4. Important Considerations
– Logging: Always implement logging in your tasks. CodeIgniter provides logging methods like `$this->log->write_log()`. This way, you can easily track if the job ran successfully or encountered errors.
– Time Limits: PHP scripts usually have a time limit. Ensure long-running tasks don’t hit this limit or consider increasing it using `set_time_limit()`.
– Database Connections: If your cron task involves a lot of database operations, make sure you don’t hit the database connection limit.
5. Advanced Examples
- a) Backing up the Database Weekly
CodeIgniter provides a Database Utility Class that makes database backups a breeze:
```php public function backup_database() { if (!$this->input->is_cli_request()) { exit('This script can only be accessed via the command line.'); } $this->load->dbutil(); $backup = $this->dbutil->backup(); $this->load->helper('file'); write_file('/path/to/backup.gz', $backup); } ```
Set it as a weekly cron job:
``` 0 0 * * 0 /usr/bin/php /path/to/your/ci/index.php reports backup_database ```
- b) Clearing Cache Monthly:
```php public function clear_cache() { if (!$this->input->is_cli_request()) { exit('This script can only be accessed via the command line.'); } $this->output->delete_cache(); } ```
Set this to run on the first day of every month:
``` 0 0 1 * * /usr/bin/php /path/to/your/ci/index.php reports clear_cache ```
Conclusion
Automating routine tasks is essential to maintain a healthy, efficient, and scalable application. Cron jobs, when combined with the flexibility of CodeIgniter, provide a robust mechanism to achieve this. Always test your cron tasks in a safe environment before deploying them to a live server, and monitor their execution regularly to ensure smooth operations. Happy automating!
Table of Contents
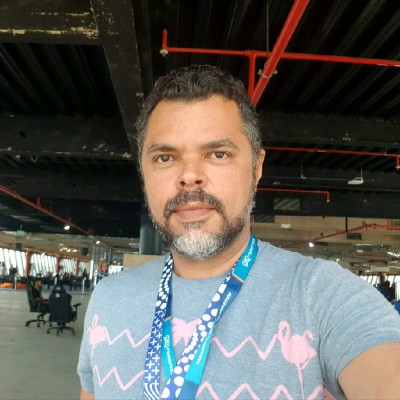
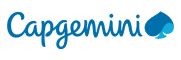