CodeIgniter and Data Encryption: Safeguarding User Information
In an age where data breaches and cyber-attacks are increasingly common, safeguarding user information is a top priority for web developers. CodeIgniter, a popular PHP framework, offers robust encryption tools that make it easier to protect sensitive data. In this blog, we’ll explore how to implement data encryption in CodeIgniter, ensuring your application’s data remains secure.
Understanding Data Encryption in CodeIgniter
Data encryption transforms readable data into an unreadable format using algorithms and a secret key. Only those who have the decryption key can convert the data back to its original form. This process is crucial for protecting sensitive information like passwords, personal data, and payment details.
CodeIgniter provides a built-in Encryption library that simplifies the encryption and decryption processes. The library supports various encryption algorithms and offers a straightforward API for developers.
Setting Up Encryption in CodeIgniter
To start using the encryption library in CodeIgniter, you need to load it in your controller. You can do this manually or autoload it by adding it to the autoload.php configuration file.
Manual Loading Example:
```php class DataController extends CI_Controller { public function __construct() { parent::__construct(); $this->load->library('encryption'); } // Other methods... } ```
Autoloading Example:
To autoload the encryption library, add it to the $autoload[‘libraries’] array in application/config/autoload.php:
```php $autoload['libraries'] = array('encryption'); ```
Configuring the Encryption Key
An encryption key is essential for the encryption process. You can set this key in the config/encryption.php file. It’s crucial to use a strong and unique key, as it’s the foundation of your data’s security.
```php $config['encryption_key'] = 'your-secret-key-here'; ```
Encrypting Data
Once the library is loaded, you can use the encrypt() method to encrypt data. This method returns an encrypted string that can be safely stored in a database or transmitted over the network.
Example:
```php $plain_text = 'Sensitive data here'; $encrypted_text = $this->encryption->encrypt($plain_text); echo $encrypted_text; ```
In this example, $plain_text contains the data you want to encrypt. The $this->encryption->encrypt() method converts it into an encrypted string, which is stored in $encrypted_text.
Decrypting Data
To retrieve the original data, use the decrypt() method. This method requires the encrypted string and the same encryption key used during the encryption process.
Example:
```php $encrypted_text = '...'; // The encrypted string $decrypted_text = $this->encryption->decrypt($encrypted_text); echo $decrypted_text; ```
The $this->encryption->decrypt() method converts the encrypted string back into its original form, stored in $decrypted_text.
Best Practices for Data Encryption
Use Strong Encryption Keys
Always use strong, unique encryption keys. Avoid hardcoding them directly in your code; instead, store them securely in environment variables or configuration files outside the web root.
Regularly Update Encryption Algorithms
As technology evolves, older encryption algorithms may become vulnerable. Regularly update your encryption methods to use the latest, most secure algorithms.
Encrypt Sensitive Data Only
While encryption is a powerful tool, it should be used judiciously. Encrypt only sensitive data to avoid unnecessary overhead and complexity.
Secure Key Management
Proper key management is critical. Ensure that encryption keys are securely stored and access is restricted to authorized personnel only.
Conclusion
Data encryption is a vital component of modern web security, providing a strong line of defense against unauthorized access to sensitive information. CodeIgniter’s built-in encryption library offers a simple yet powerful way to implement encryption in your applications. By following best practices and keeping your encryption methods up-to-date, you can ensure that your users’ data remains safe and secure.
Further Reading
Table of Contents
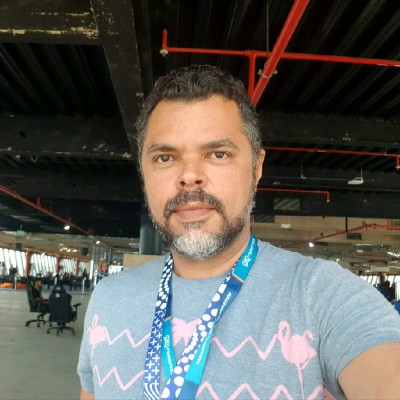
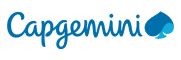