Mastering User Data Handling with CodeIgniter Session Management
Session management is a crucial part of any web application, and proficiently leveraging it can require skilled professionals. This is where the expertise of CodeIgniter developers comes into play. CodeIgniter developers can adeptly manage the system to track interactions and data exchange between users and the application, creating unique user experiences and personalized interfaces. CodeIgniter, a powerful PHP framework with a tiny footprint, offers an easy and effective way to manage sessions, especially when navigated by proficient developers.
In this blog post, we will explore how to handle user data using CodeIgniter session management. We’ll look into setting up sessions, storing and retrieving user data, and dealing with other session-related tasks. Let’s get started.
Understanding CodeIgniter Session
A session in CodeIgniter is a way of storing user-specific data to be used across multiple pages or scripts. This information is stored on the server-side, making it secure and efficient. CodeIgniter’s session library offers advanced features such as session encryption, flashdata (session data that will only be available for the next server request), and tempdata (session data with a specific lifespan).
Setting up CodeIgniter Session
The first step in using CodeIgniter sessions is to load the Session library. This can be done in two ways. You can autoload it by updating the `application/config/autoload.php` file:
```php $autoload['libraries'] = array('database', 'session');
Or you can manually load the Session library when needed in your controller:
```php $this->load->library('session');
After loading the Session library, we can use its methods and properties through the `$this->session` object.
Storing User Data in CodeIgniter Session
Storing data in CodeIgniter session involves setting session data using the `set_userdata` method. Here’s a simple example:
```php $userData = array( 'username' => 'John Doe', 'email' => 'johndoe@example.com', 'logged_in' => TRUE ); $this->session->set_userdata($userData);
In the example above, we stored an array of user data into the session. We can also store individual key-value pairs:
```php $this->session->set_userdata('username', 'John Doe');
Retrieving User Data from CodeIgniter Session
Retrieving user data from a session is just as straightforward. Use the `userdata` method and provide the key of the data you want to retrieve:
```php $username = $this->session->userdata('username');
If you want to get all session data, simply call the `userdata` method without any arguments:
```php $all_userdata = $this->session->userdata();
Checking If Session Data Exists
Before attempting to retrieve session data, it’s good practice to check if the data exists. We can do this with the `has_userdata` function:
```php if($this->session->has_userdata('username')) { $username = $this->session->userdata('username'); }
Removing User Data from Session
Removing data from the session can be done using the `unset_userdata` function. Like the `set_userdata` function, this function can also accept an array or a single key:
```php // Removing a single item $this->session->unset_userdata('username'); // Removing multiple items $userData = array('username', 'email'); $this->session->unset_userdata($userData);
Destroying a Session
If you want to destroy the entire session, you can use the `sess_destroy` method:
```php $this->session->sess_destroy();
This is useful when users log out of the system, ensuring that no sensitive data remains in the session.
Flashdata: Temporarily Storing User Data
Flashdata in CodeIgniter is a type of session data that will only be available for the next server request and is then automatically cleared. This feature is useful for storing temporary messages like form validation messages or one-time notification alerts.
Here’s how you can set flashdata:
```php $this->session->set_flashdata('item', 'value');
To retrieve flashdata, use the `flashdata` function:
```php $this->session->flashdata('item');
Tempdata: Session Data with a Lifespan
Tempdata is another type of session data that has a specific lifespan. After the lifespan has expired, the data is automatically removed.
Here’s an example of setting tempdata:
```php // This data will be cleared after 300 seconds (5 minutes) $this->session->set_tempdata('item', 'value', 300);
You can retrieve tempdata in the same way you would retrieve any session data:
```php $this->session->tempdata('item');
Conclusion
CodeIgniter’s Session library, when utilized by skilled CodeIgniter developers, provides a simple and secure way to manage user data across multiple page requests. With its built-in support for storing different types of session data such as normal, flashdata, and tempdata, you have complete control over how and when your data should be stored and cleared.
The expertise of CodeIgniter developers becomes particularly valuable in using session data appropriately, as this is crucial in creating secure, interactive, and personalized experiences for your users. Understanding the features and capabilities provided by CodeIgniter session management can dramatically improve your web application, making a compelling case to hire CodeIgniter developers for managing your project.
Table of Contents
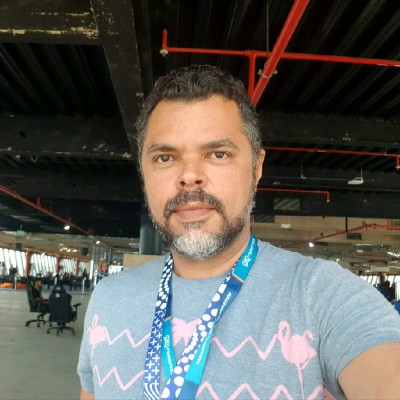
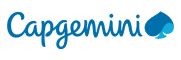