What is CodeIgniter’s database caching and how does it work?
CodeIgniter’s database caching is a powerful feature that allows you to improve the performance of database-driven applications by storing query results in a cache for subsequent use. This feature helps reduce the load on the database server and speeds up the retrieval of data.
Here’s how CodeIgniter’s database caching works:
- Caching Configuration: To enable database caching in CodeIgniter, you need to configure it in your application’s configuration file (`config/database.php`). You can set the caching settings for your database connection by specifying parameters like ‘cache_on’ and ‘cachedir’. For example:
```php $db['default'] = array( 'dsn' => '', 'hostname' => 'localhost', 'username' => 'your_username', 'password' => 'your_password', 'database' => 'your_database', 'dbdriver' => 'mysqli', 'cache_on' => TRUE, // Enable caching 'cachedir' => APPPATH . 'cache/db_cache/', // Specify the cache directory ); ```
- Query Caching: Once caching is enabled, you can specify which database queries you want to cache by using the `$this->db->cache_on()` method before executing a query. For example:
```php $this->db->cache_on(); // Enable caching for the next query $query = $this->db->get('your_table'); ```
This query result will be stored in the cache directory you specified.
- Cache Expiration: You can set an expiration time for cached query results to ensure that stale data doesn’t get served. This is done using the `$this->db->cache_off()` method with an optional expiration time in seconds:
```php $this->db->cache_off(3600); // Cache expires after 1 hour (3600 seconds) ```
- Query Result Retrieval: When you execute a query that has caching enabled, CodeIgniter will first check if the result is already cached. If it is, the cached data is returned directly instead of hitting the database server. If not, the query is executed, and the result is stored in the cache for future use.
- Cache Clearing: To clear the database query cache, you can use the `$this->db->cache_delete()` method with a table name or a custom cache key:
```php $this->db->cache_delete('your_table'); // Clears cache for a specific table ```
CodeIgniter’s database caching is a valuable tool for optimizing database-driven applications, especially when dealing with frequently accessed data. By intelligently using caching, you can reduce database load, decrease response times, and improve overall application performance. However, it’s essential to manage cache expiration and clearing carefully to ensure data accuracy and consistency.
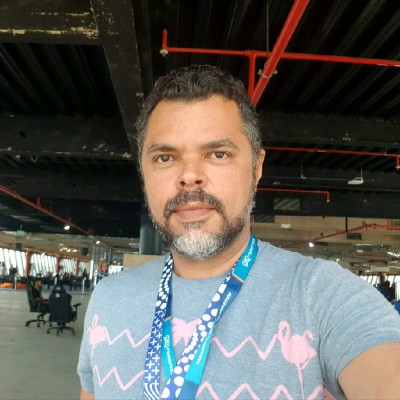
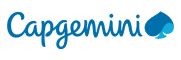