Elevate Your CodeIgniter Projects with Dependency Injection Techniques
Dependency Injection (DI) is a software design pattern that allows us to inject dependencies (services or objects that a class needs) instead of a class creating them internally. It promotes a more maintainable, testable, and modular code base. CodeIgniter, a popular PHP framework, does not include a built-in DI container by default like some other frameworks do, but it does provide the flexibility to integrate one or implement DI practices manually.
In this post, we’ll explore how to manage complex application dependencies in CodeIgniter through Dependency Injection.
1. Why Dependency Injection?
Before we dive into DI in CodeIgniter, let’s understand why DI is a valuable addition:
- Testability: With DI, you can easily mock your dependencies, making unit testing more straightforward.
- Flexibility: By decoupling the dependencies, you can switch or update individual components without changing the dependent class.
- Maintainability: By avoiding tight coupling between components, the code becomes easier to maintain and modify.
2. Implementing Dependency Injection in CodeIgniter
2.1. Manual Dependency Injection
Though not fancy, the simplest way to implement DI is to manually inject dependencies through constructors or setter methods.
```php class OrderService { protected $repository; public function __construct(OrderRepository $repository) { $this->repository = $repository; } } // Usage $repository = new OrderRepository(); $orderService = new OrderService($repository); ```
In the above example, instead of the `OrderService` class internally creating an instance of `OrderRepository`, we inject it from the outside.
2.2. Service Container Approach
For larger applications, manually wiring up dependencies might become cumbersome. Service containers can manage the instantiation and sharing of objects.
While CodeIgniter doesn’t have a built-in service container, we can use third-party containers like PHP-DI or Pimple.
Using PHP-DI with CodeIgniter:
First, install PHP-DI via Composer:
```bash composer require php-di/php-di ```
Then, integrate it with CodeIgniter:
```php // application/config/container.php use DI\ContainerBuilder; $builder = new ContainerBuilder(); $builder->addDefinitions([ 'OrderRepository' => \DI\create('OrderRepository'), 'OrderService' => \DI\autowire('OrderService') ]); return $builder->build(); ```
Now, you can fetch and use the services:
```php $container = require APPPATH . 'config/container.php'; $orderService = $container->get('OrderService'); ```
With PHP-DI’s autowiring, it automatically resolves the dependencies of the `OrderService`.
3. Advanced Dependency Management
When dependencies become complex, managing them requires more thought:
- Lazy Loading: Instead of initializing all dependencies upfront, initialize them only when they’re needed. Containers like PHP-DI support lazy loading out of the box.
- Configuration and Environment-based Dependencies: Sometimes, the dependency you want to inject can vary based on configuration or environment. A container can help manage these dynamic dependencies.
```php // application/config/container.php use DI\ContainerBuilder; $builder = new ContainerBuilder(); $builder->addDefinitions([ 'DatabaseConnection' => function() { $config = require APPPATH . 'config/database.php'; if (ENVIRONMENT === 'production') { return new ProductionDatabaseConnection($config['production']); } return new DevelopmentDatabaseConnection($config['development']); }, ]); return $builder->build(); ```
- Decorators: If you need to alter or add behavior to a service without modifying the service itself, use decorators. Inject the original service into the decorator and override the specific methods.
```php class LoggingOrderServiceDecorator { private $orderService; private $logger; public function __construct(OrderService $orderService, Logger $logger) { $this->orderService = $orderService; $this->logger = $logger; } public function placeOrder($orderData) { $this->logger->info("Placing order", $orderData); $this->orderService->placeOrder($orderData); } } ```
Conclusion
Dependency Injection helps in creating a flexible and maintainable application. Even though CodeIgniter doesn’t provide an out-of-the-box DI solution, its flexibility allows you to implement manual DI or integrate with third-party DI containers. As application dependencies grow in complexity, strategies like lazy loading, environment-based dependencies, and decorators can be leveraged to maintain a clean and organized codebase.
In the world of evolving application architectures, embracing patterns like Dependency Injection ensures that our applications remain robust, testable, and adaptable to change.
Table of Contents
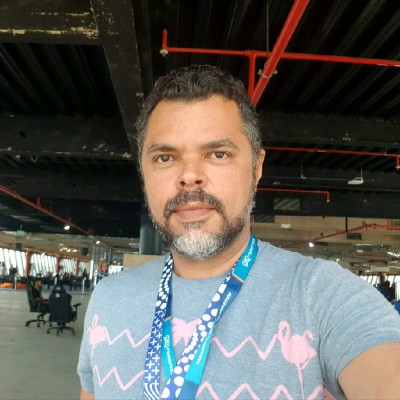
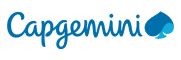