Elasticsearch Meets CodeIgniter: The Future of Advanced Web App Search
When it comes to building modern web applications, search capabilities are not just a luxury but a necessity. While CodeIgniter provides a solid foundation for web app development, integrating advanced search features may require some external assistance. Enter Elasticsearch, a powerful, full-text search and analytics engine that can seamlessly complement CodeIgniter. In this blog post, we will explore how to integrate Elasticsearch with a CodeIgniter application and implement advanced search functionalities.
1. Why Choose Elasticsearch?
Before diving into the implementation, it’s essential to understand why Elasticsearch stands out:
- Scalability: Elasticsearch is designed to be scalable right from the start. As your data grows, you can scale out your clusters.
- Full-text Search: Elasticsearch offers a powerful full-text search engine with support for complex query types, such as wildcard, fuzzy search, and more.
- Real-time Indexing: Any changes made to the data get indexed immediately, making the updated data searchable in near real-time.
2. Setting Up Elasticsearch
To start with, you’ll need to install Elasticsearch. You can refer to the official [Elasticsearch installation guide](https://www.elastic.co/guide/en/elasticsearch/reference/current/install-elasticsearch.html) for detailed steps.
3. Integrating with CodeIgniter
Once Elasticsearch is up and running, the next step is to integrate it with your CodeIgniter application.
- Installing Elasticsearch Client for PHP:
You can use Composer to install the official Elasticsearch client for PHP:
``` composer require elasticsearch/elasticsearch ```
- Setting Up the Configuration:
Create a new configuration file at `application/config/elasticsearch.php`:
```php <?php $config['es_host'] = 'localhost'; $config['es_port'] = '9200'; $config['es_index'] = 'my_index'; ```
- Initializing the Client:
You can load the configuration and initialize the Elasticsearch client in your controller or model:
```php $this->load->config('elasticsearch'); $esClient = \Elasticsearch\ClientBuilder::create() ->setHosts([$this->config->item('es_host') . ':' . $this->config->item('es_port')]) ->build(); ```
4. Adding Data to Elasticsearch
To search for data, you first need to index it. Let’s take an example of adding a blog post:
```php $data = [ 'index' => $this->config->item('es_index'), 'id' => $post_id, 'body' => [ 'title' => $title, 'content' => $content, 'date' => $date ] ]; $response = $esClient->index($data); ```
5. Implementing the Search
Now for the fun part! Here’s a basic example of implementing a search for blog posts:
```php $searchParams = [ 'index' => $this->config->item('es_index'), 'body' => [ 'query' => [ 'match' => [ 'title' => $search_query ] ] ] ]; $results = $esClient->search($searchParams); ```
For more complex searches, you can utilize features like:
– Wildcard Searches: This allows you to search for partial words. For instance, `ti*` would match titles like “tiger” and “tide.”
– Fuzzy Searches: Useful for handling typos. A search for “appel” can still match “apple”.
– Boolean Queries: Combine multiple queries, for example, to search for posts that contain “apple” but not “orange”.
6. Advancing the Implementation
Elasticsearch provides a plethora of functionalities you can leverage:
- Faceted Search: Allows you to classify search results into categories based on certain criteria.
- Highlighting: Highlights the search terms in the results.
- Synonym Handling: For example, you can make “cellphone” and “mobile” synonymous in searches.
Conclusion
Incorporating Elasticsearch into your CodeIgniter application not only provides advanced search capabilities but also offers a scalable solution for large datasets. While the examples here give a foundational idea, diving deeper into Elasticsearch’s documentation will unlock its full potential. Whether you’re building a content-rich website, an e-commerce platform, or any application where search plays a pivotal role, integrating CodeIgniter with Elasticsearch is a step in the right direction.
Table of Contents
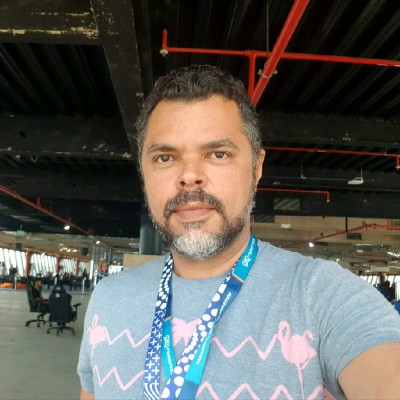
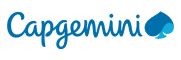