What is CodeIgniter’s encryption library, and how can it be used for securing data?
CodeIgniter’s encryption library is a powerful tool that allows you to secure sensitive data within your web applications by encrypting and decrypting it. It employs various encryption algorithms and methods to protect data during transmission and storage. Here’s how you can use CodeIgniter’s encryption library for securing data:
- Library Initialization:
To begin using the encryption library, you need to load it in your controller or model using the following code:
```php $this->load->library('encryption'); ```
- Configuration:
CodeIgniter provides a configuration file (`encryption.php`) where you can set encryption preferences, including the encryption driver, encryption key, and other settings. It’s essential to configure this file before using the encryption library.
- Encryption and Decryption:
You can use the `encrypt()` method to encrypt data and the `decrypt()` method to decrypt it. For example:
```php $plainText = 'Sensitive Data'; $encryptedData = $this->encryption->encrypt($plainText); $decryptedData = $this->encryption->decrypt($encryptedData); ```
Ensure that you store the encrypted data securely, as the decryption process requires the appropriate key and configuration.
- Encryption Key Management:
The encryption library relies on a secret encryption key for its operations. It’s crucial to keep this key secure. You can set the key in the `encryption.php` configuration file or dynamically load it from a secure location.
- Using Encryption in Applications:
Encrypt sensitive user data such as passwords, API tokens, or any confidential information before storing it in your database. When transmitting data over unsecured channels like HTTP, consider encrypting the data to protect it from eavesdropping.
- Data Integrity:
Encryption not only secures data but also ensures its integrity. Any tampering with encrypted data will result in decryption failure, alerting you to potential security breaches.
- Error Handling:
Implement proper error handling when using the encryption library. Incorrect keys or configuration can lead to data loss or corruption.
- Testing:
Thoroughly test your encryption and decryption processes to ensure they work as expected. Simulate different scenarios to verify the security of your application.
- Documentation and Compliance:
Clearly document your encryption practices and ensure they comply with relevant security standards and regulations, such as GDPR or HIPAA.
By using CodeIgniter’s encryption library, you can enhance the security of your application and protect sensitive data from unauthorized access, providing a safer experience for your users.
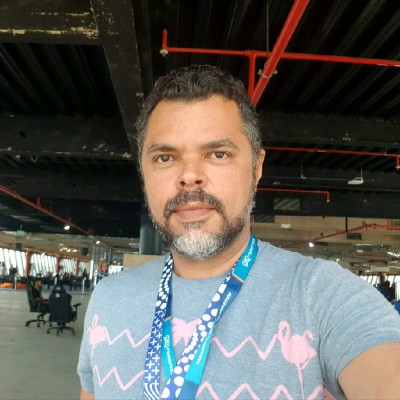
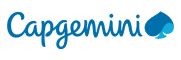