Transform Your Web Projects with Advanced File Handling in CodeIgniter
CodeIgniter, an open-source software rapid development web framework, has always been a preferred choice among developers for its simplicity, speed, and ease of use. For businesses looking to make the most out of this framework, it’s often a smart choice to hire CodeIgniter developers. One of the many features that these developers frequently utilize is the file uploading library. The ability to handle file uploads securely and efficiently is a crucial part of many web applications today. In this blog post, we will delve into how to work with CodeIgniter’s File Upload library and tackle file manipulations effectively.
Table of Contents
1. Getting Started with CodeIgniter’s File Upload Library
To use the File Upload library, it must first be initialized. You can do this either by autoload configuration or manually within your controller.
Autoload Configuration:
```php $autoload['libraries'] = array('upload'); ```
Manually within your Controller:
```php $this->load->library('upload'); ```
2. Basic File Upload
Configurations:
Before proceeding with the actual upload process, set your upload preferences.
```php $config['upload_path'] = './uploads/'; // Directory to save the uploaded files $config['allowed_types'] = 'gif|jpg|png'; // Allowed file types $config['max_size'] = 100; // File size limit (in KB) $config['max_width'] = 1024; // Maximum image width (in pixels) $config['max_height'] = 768; // Maximum image height (in pixels) $this->load->library('upload', $config); ```
Uploading a File:
Once configurations are set, the next step is to attempt the upload:
```php if (!$this->upload->do_upload('userfile')) { $error = array('error' => $this->upload->display_errors()); $this->load->view('upload_form', $error); } else { $data = array('upload_data' => $this->upload->data()); $this->load->view('upload_success', $data); } ```
Here, `’userfile’` is the default form field name. This can be changed as per your requirement.
3. File Manipulation: Image Resizing
Once you have uploaded a file, especially images, you might want to perform some manipulations, such as resizing. CodeIgniter provides an Image Manipulation Class to assist with tasks like these.
Configurations:
```php $config['image_library'] = 'gd2'; $config['source_image'] = '/path/to/image.jpg'; $config['create_thumb'] = TRUE; $config['maintain_ratio'] = TRUE; $config['width'] = 75; $config['height'] = 50; $this->load->library('image_lib', $config); $this->image_lib->resize(); ```
This will resize the image and create a thumbnail for the same.
4. File Manipulation: Image Rotation
If you wish to rotate an uploaded image:
Configurations:
```php $config['image_library'] = 'gd2'; $config['source_image'] = '/path/to/image.jpg'; $config['rotation_angle'] = 'hor'; $this->load->library('image_lib', $config); if (!$this->image_lib->rotate()) { echo $this->image_lib->display_errors(); } ```
5. File Manipulation: Image Cropping
Cropping an image is also a simple task using CodeIgniter’s Image Manipulation Class:
Configurations:
```php $config['image_library'] = 'gd2'; $config['source_image'] = '/path/to/image.jpg'; $config['x_axis'] = '100'; $config['y_axis'] = '60'; $this->load->library('image_lib', $config); if (!$this->image_lib->crop()) { echo $this->image_lib->display_errors(); } ```
6. Tips for Handling File Uploads in CodeIgniter
- Validation: Ensure that you validate the uploaded file type and size before processing it. This will safeguard your application against potential malicious files.
- File Naming: Use unique naming conventions for your uploaded files to avoid accidental overwriting of existing files.
- Security: Never trust the user’s input. Always sanitize and verify file inputs, and make sure you have proper security measures in place, such as setting the correct file permissions.
Conclusion
Handling file uploads and manipulations in CodeIgniter is a breeze, thanks to its comprehensive libraries. With minimal configuration, developers can perform complex tasks like uploading, resizing, rotating, and cropping with ease. Always remember to keep security best practices in mind when working with file uploads to keep your applications safe and robust.
Whether you’re building a photo gallery, a content management system, or any web application requiring file handling, CodeIgniter’s File Upload and Image Manipulation libraries will undoubtedly be essential tools in your developer toolkit. If you’re looking to harness the full potential of these features, consider hiring CodeIgniter developers to optimize your project.
Table of Contents
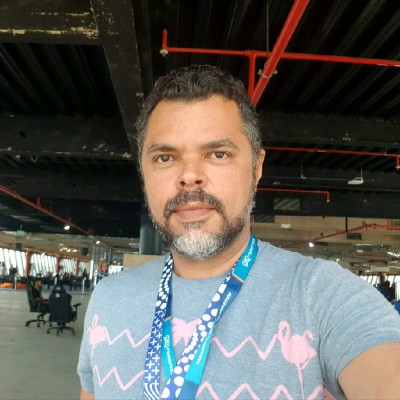
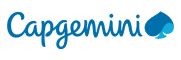