Want Real-time Capabilities? Here’s Your Guide to CodeIgniter and Firebase
CodeIgniter, a robust PHP framework, and Firebase, a Google-backed Real-time Database, make for an impressive duo when it comes to creating real-time applications. By integrating both technologies, developers can enjoy the quick development cycles of CodeIgniter with the real-time capabilities of Firebase.
In this post, we’ll walk you through how to synchronize data in real-time and set up notifications using CodeIgniter and Firebase.
1. Setting up Firebase in Your CodeIgniter Project
Before you start, make sure you have a Firebase project set up. If not, head over to the Firebase console, create a new project, and grab your API credentials.
Step 1: Inside your CodeIgniter project directory, install Firebase PHP SDK using Composer:
```bash composer require kreait/firebase-php ```
Step 2: In your `application/config` directory, create a new config file named `firebase.php`. Inside this file, add:
```php <?php defined('BASEPATH') OR exit('No direct script access allowed'); $config['firebase_config'] = [ 'projectId' => 'YOUR_PROJECT_ID', 'keyFile' => 'path_to_your_service_account_json_file.json', // Change the path accordingly ]; ```
Step 3: In your CodeIgniter controller or model, initialize the Firebase SDK:
```php $this->load->config('firebase'); $factory = (new \Kreait\Firebase\Factory())->withServiceAccount($this->config->item('keyFile', 'firebase_config')); $database = $factory->createDatabase(); ```
2. Real-time Data Syncing with CodeIgniter and Firebase
Imagine you want to sync user registration data. Here’s how you can push new user data from CodeIgniter to Firebase in real-time.
```php // Assuming you have user data in $userData array $userRef = $database->getReference('users/' . $userData['id']); $userRef->set($userData); ```
To listen for changes in Firebase and fetch data in real-time, Firebase Web SDK would be more suitable. Include the SDK in your view:
```javascript <script src="https://www.gstatic.com/firebasejs/8.0.0/firebase-app.js"></script> <script src="https://www.gstatic.com/firebasejs/8.0.0/firebase-database.js"></script> <script> // TODO: Replace with your project's customized code snippet var config = { apiKey: "YOUR_API_KEY", authDomain: "YOUR_AUTH_DOMAIN", databaseURL: "YOUR_DATABASE_URL", projectId: "YOUR_PROJECT_ID", }; firebase.initializeApp(config); var usersRef = firebase.database().ref('users'); usersRef.on('value', function(snapshot) { console.log(snapshot.val()); }); </script> ```
3. Real-time Notifications with Firebase
Using Firebase’s Cloud Messaging, you can send notifications to users. Here’s a basic example of how to set it up:
Step 1: On the Firebase Console, navigate to Cloud Messaging and get your server key.
Step 2: In `firebase.php`, add the server key:
```php $config['firebase_messaging_server_key'] = 'YOUR_SERVER_KEY'; ```
Step 3: To send a notification:
```php $messaging = $factory->createMessaging(); $message = \Kreait\Firebase\Messaging\CloudMessage::fromArray([ 'token' => 'RECIPIENT_TOKEN', 'notification' => ['title' => 'Title', 'body' => 'Notification body'] ]); $messaging->send($message); ```
For the client side, integrate the Firebase JS SDK to receive notifications. Remember to request permission from users to send them notifications.
4. Benefits of this Integration
– Real-time Capabilities: Firebase provides real-time data syncing, which means any data changes are instantly reflected across all clients.
– Scalability: Firebase is a managed service that scales automatically as your user base grows.
– Rapid Development: Combining the rapid development benefits of CodeIgniter with Firebase’s real-time features results in faster product launches.
5. Limitations and Considerations
– Cost: Firebase’s real-time database and messaging might become costly as usage grows.
– Learning Curve: While Firebase is easy to start with, mastering its various features can be challenging.
Conclusion
Combining CodeIgniter and Firebase offers a robust solution for applications that require real-time data synchronization and messaging. While there are costs and a learning curve to consider, the benefits of rapid development, scalability, and real-time capabilities make it a powerful combination for modern web applications.
Remember to keep an eye on costs as your user base grows and to properly secure your Firebase data using security rules. Happy coding!
Table of Contents
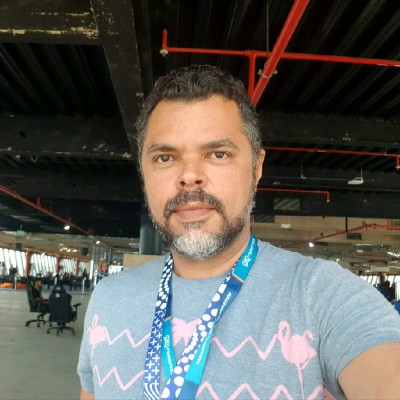
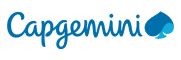