Say Goodbye to Complex Forms: Embrace CodeIgniter’s Form Helper Today
CodeIgniter, a powerful PHP framework, offers developers a plethora of tools and utilities that simplify web application development. For those who want to harness its full potential, there’s an increasing demand to hire CodeIgniter developers. Among the most useful utilities is the Form Helper, which assists in creating and validating form elements effortlessly. In this blog post, we’ll delve into how the CodeIgniter Form Helper can simplify form creation, accompanied by practical examples.
Table of Contents
1. Introduction to CodeIgniter’s Form Helper
Every web developer understands the challenge of creating forms. From maintaining consistency to handling user input securely, a lot of considerations go into designing and implementing a web form. CodeIgniter’s Form Helper provides a set of functions that eliminate a lot of the HTML markup necessary for creating forms.
To use the Form Helper, it must first be loaded:
```php $this->load->helper('form'); ```
2. Basic Form Creation
Once the Form Helper is loaded, you can generate form elements with ease. Let’s start with a simple example:
Without Form Helper:
```html <form action="action_page.php" method="post"> <input type="text" name="username"> <input type="submit" value="Submit"> </form> ```
With Form Helper:
```php echo form_open('action_page.php'); echo form_input('username'); echo form_submit('submit', 'Submit'); echo form_close(); ```
The above code snippets achieve the same result. However, using the Form Helper ensures that your forms are more consistent and reduces manual HTML markup.
3. Creating Dropdowns and Textareas
Dropdowns and text areas are frequently used form components. Let’s see how the Form Helper aids in their creation.
Dropdown:
```php $options = array( 'small' => 'Small Shirt', 'med' => 'Medium Shirt', 'large' => 'Large Shirt', 'xlarge' => 'Extra Large Shirt', ); $selected = array('med'); echo form_dropdown('shirts', $options, $selected); ```
Textarea:
```php $data = array( 'name' => 'message', 'rows' => '5', 'cols' => '10', 'value' => 'Default Text', ); echo form_textarea($data); ```
4. Setting Default Values
Default values can be set for form elements, ensuring that they are populated even if the user doesn’t provide input.
```php echo form_input('username', 'Default Username'); ```
5. Using Form Validation
CodeIgniter not only helps in form creation but also provides built-in validation tools.
To use the form validation library, load it:
```php $this->load->library('form_validation'); ```
Set validation rules:
```php $this->form_validation->set_rules('username', 'Username', 'required'); $this->form_validation->set_rules('password', 'Password', 'required|min_length[8]'); ```
And finally, check if the form is valid:
```php if ($this->form_validation->run() == FALSE) { // There are errors echo validation_errors(); } else { // Form is valid // Handle the form data } ```
6. Populating Forms from Databases
Often, forms need to be populated from database data, especially for tasks like editing records. CodeIgniter’s Form Helper allows easy binding of form fields to database query results.
Assuming you’ve fetched a user record as `$user`:
```php echo form_input('username', $user->username); ```
This will populate the ‘username’ input with the `username` property from the `$user` object.
7. Handling File Uploads
To handle file uploads, you can use CodeIgniter’s File Uploading Class alongside the Form Helper.
```php echo form_open_multipart('upload/do_upload'); echo form_upload('userfile'); echo form_submit('upload', 'Upload'); echo form_close(); ```
Closing Remarks
The CodeIgniter Form Helper, as seen from the examples above, brings simplicity and efficiency to form creation and validation. Instead of manually writing out each HTML tag and attribute, developers can leverage the Form Helper’s methods for more concise, readable, and maintainable code.
As web forms remain an integral part of user interaction in web applications, mastering tools that simplify form creation can save considerable development time and reduce errors. For businesses looking to optimize this process further, it might be beneficial to hire CodeIgniter developers. Whether you’re new to CodeIgniter or an experienced developer, make sure to utilize its Form Helper to get the best out of your form-based functionalities.
Table of Contents
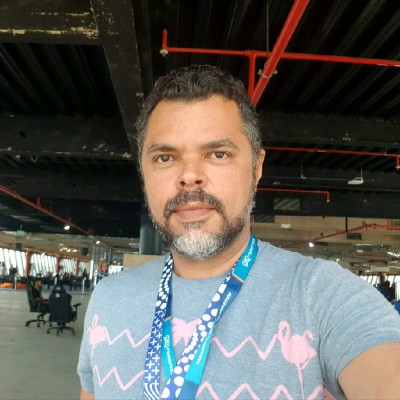
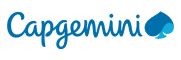