How to use CodeIgniter’s form validation library for complex validation rules?
CodeIgniter’s form validation library is a robust tool that allows you to validate user input effectively, ensuring that data submitted to your web application meets specific criteria and adheres to your defined rules. While it’s excellent for basic validation, it’s equally capable of handling complex validation rules. Here’s how you can use CodeIgniter’s form validation library for complex validation scenarios:
- Load the Form Validation Library:
To get started, you need to load the Form Validation library in your controller. You can do this in the constructor or any method where you plan to use it.
```php $this->load->library('form_validation'); ```
- Set Validation Rules:
Define your validation rules using the `set_rules()` method. For complex rules, you can use callback functions. Callbacks allow you to write custom validation methods in your controller.
```php $this->form_validation->set_rules('email', 'Email', 'required|valid_email|callback_custom_validation'); ```
- Create Custom Validation Methods:
To handle complex validation, define custom callback methods in your controller. These methods should perform the validation logic and return a Boolean value indicating whether the input is valid or not.
```php public function custom_validation($str) { // Complex validation logic here if (/* Your condition */) { return true; // Valid } else { $this->form_validation->set_message('custom_validation', 'The {field} field is not valid.'); return false; // Invalid } } ```
- Perform Validation:
In your controller method where you handle the form submission, call the `run()` method to perform the validation. It will return `true` if all rules pass, or `false` otherwise.
```php if ($this->form_validation->run() === false) { // Validation failed, handle errors } else { // Validation passed, proceed with data processing } ```
- Display Validation Errors:
If validation fails, CodeIgniter will automatically populate an array of error messages. You can retrieve and display these messages in your view to inform users about the errors.
```php echo validation_errors(); ```
By using custom callback methods and the flexibility of the Form Validation library, you can tackle complex validation scenarios effectively. Whether it’s comparing fields, validating against external data sources, or performing intricate business logic checks, CodeIgniter’s form validation library can handle it all. This approach ensures that your application maintains data integrity and provides a seamless user experience by guiding users to submit correct and valid information.
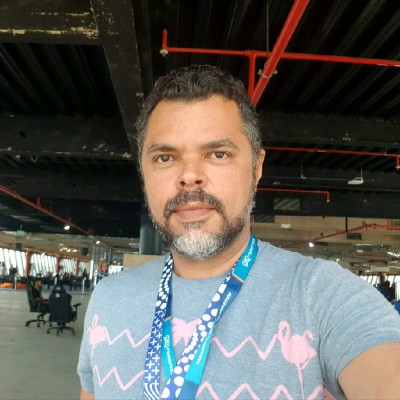
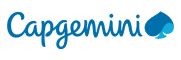