Maximizing Web Interaction: Integrate Google Maps with CodeIgniter Today
Location-based services are at the forefront of today’s web applications, providing added value to users through interactive maps, location tracking, and geographical data. CodeIgniter, a popular PHP framework, and the Google Maps API, a robust mapping service, can be combined to seamlessly incorporate these features into web apps. In this post, we’ll dive into how you can leverage both technologies to enhance your projects.
Setting the Stage: Basics of CodeIgniter and Google Maps API
CodeIgniter: A powerful PHP framework with a lean footprint, it is designed for developers who need a toolkit to create full-featured web applications. It offers libraries for connecting to the database, sending emails, form validation, session management, and more.
Google Maps API: A collection of APIs that allow developers to embed Google Maps on web pages using JavaScript or Flash. Features include placing markers on the map, plotting routes, and generating dynamic location data.
1. Setting Up CodeIgniter
Download and install CodeIgniter by following instructions from the official website. Once set up, your directory structure should resemble:
``` /application /system /index.php /assets (optional folder for JavaScript, CSS, etc.) ```
2. Integrating Google Maps API
You’ll need an API key to start using Google Maps. Head over to the Google Cloud Platform Console to create a project and enable the Maps JavaScript API. After enabling, you’ll receive an API key which we will use shortly.
Displaying a Basic Map
In your CodeIgniter `assets` folder, create a new JS file, `map.js`. Add the following code:
```javascript function initMap() { var mapOptions = { center: {lat: -34.397, lng: 150.644}, zoom: 8 }; var map = new google.maps.Map(document.getElementById("map"), mapOptions); } ```
Next, in your view (for instance, `application/views/map_view.php`):
```html <!DOCTYPE html> <html> <head> <title>CodeIgniter & Google Maps</title> <script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap" async defer></script> <script src="<?php echo base_url('assets/map.js'); ?>"></script> </head> <body> <div id="map" style="width:500px;height:400px;"></div> </body> </html> ```
Remember to replace `YOUR_API_KEY` with the key you got from the Google Cloud Platform.
Adding a Marker to the Map
Enhance the `initMap` function in `map.js`:
```javascript function initMap() { var mapOptions = { center: {lat: -34.397, lng: 150.644}, zoom: 8 }; var map = new google.maps.Map(document.getElementById("map"), mapOptions); var marker = new google.maps.Marker({ position: {lat: -34.397, lng: 150.644}, map: map, title: 'Hello World!' }); } ```
Getting the User’s Location
Modify the `initMap` function further:
```javascript function initMap() { if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(function(position) { var pos = { lat: position.coords.latitude, lng: position.coords.longitude }; var map = new google.maps.Map(document.getElementById("map"), { center: pos, zoom: 15 }); var marker = new google.maps.Marker({ position: pos, map: map, title: 'Your Location' }); }, function() { handleLocationError(true, infoWindow, map.getCenter()); }); } else { // Browser doesn't support Geolocation handleLocationError(false, infoWindow, map.getCenter()); } } function handleLocationError(browserHasGeolocation, infoWindow, pos) { infoWindow.setPosition(pos); infoWindow.setContent(browserHasGeolocation ? 'Error: The Geolocation service failed.' : 'Error: Your browser doesn\'t support geolocation.'); infoWindow.open(map); } ```
Wrapping Up
Combining the power of CodeIgniter and the Google Maps API allows developers to create rich, location-aware applications. From simply displaying a map, marking points of interest, or even locating users in real-time, the opportunities are vast.
Incorporate these features into your next web application project to add value for your users. And remember, with great power comes great responsibility—always use geolocation features with privacy in mind, ensuring users are aware of and have consented to any data collection.
Table of Contents
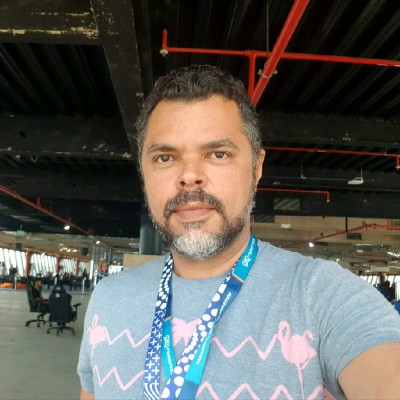
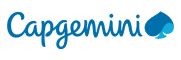