Transform Your Web Apps with CodeIgniter and GraphQL Integration
In today’s digital landscape, building scalable and efficient APIs is vital for any online application. While REST APIs have dominated the web for quite some time, GraphQL has emerged as a revolutionary technology offering more flexibility and efficiency. In this article, we will explore the power of combining CodeIgniter, a popular PHP framework, with GraphQL to build efficient APIs.
1. What is GraphQL?
GraphQL is a query language for your API, and a runtime for executing those queries using a type system you define for your data. It isn’t tied to any specific database or storage engine. Instead, it’s backed by your existing code and data.
Key benefits of GraphQL include:
- Fetch Exactly What You Need: Clients can request only the data they need, making responses faster and lighter.
- Discoverable Schema: With a strong type system, GraphQL API descriptions are clear and easy to read.
- Single Endpoint: Unlike REST where you might have multiple endpoints for different resources, in GraphQL you usually expose a single endpoint for all interactions.
2. Integrating GraphQL with CodeIgniter
To get started, you first need to have a CodeIgniter application set up. If you’re not familiar with this, you can check out the official [CodeIgniter documentation](https://codeigniter.com/user_guide/).
Once you have your CodeIgniter application, integrating GraphQL is a straightforward process.
2.1. Installing the necessary packages
To integrate GraphQL with CodeIgniter, you can use the [webonyx/graphql-php](https://github.com/webonyx/graphql-php) package. Install it using composer:
```bash composer require webonyx/graphql-php ```
2.2. Setting up a GraphQL controller
Create a new controller, `Graphql.php`, in your CodeIgniter controllers directory.
```php <?php use GraphQL\GraphQL; use GraphQL\Type\Schema; use GraphQL\Type\Definition\Type; class Graphql extends CI_Controller { public function index() { // Your GraphQL schema and resolvers will be defined here. // For now, let's keep it simple. } } ```
2.3. Define a simple schema
For demonstration, let’s create a simple query to fetch a user by ID.
```php $userType = new ObjectType([ 'name' => 'User', 'fields' => [ 'id' => Type::string(), 'name' => Type::string(), 'email' => Type::string(), ], ]); $queryType = new ObjectType([ 'name' => 'Query', 'fields' => [ 'user' => [ 'type' => $userType, 'args' => [ 'id' => Type::nonNull(Type::string()) ], 'resolve' => function ($root, $args) { // Fetch the user from your database using $args['id'] // For simplicity, we'll return a static user return [ 'id' => '1', 'name' => 'John Doe', 'email' => 'john.doe@example.com' ]; } ], ], ]); $schema = new Schema([ 'query' => $queryType ]); ```
2.4. Handling the GraphQL queries
In the `index` method of our GraphQL controller, process incoming queries.
```php public function index() { try { $input = file_get_contents('php://input'); $data = json_decode($input, true); $query = $data['query']; $result = GraphQL::executeQuery($schema, $query)->toArray(); echo json_encode($result); } catch (Exception $e) { echo json_encode(['error' => $e->getMessage()]); } } ```
Now, you have a basic GraphQL API setup using CodeIgniter. You can test the API by sending a POST request to the `/graphql` endpoint with a query.
Example request:
```json { "query": "{ user(id: \"1\") { name, email } }" } ```
Expected response:
```json { "data": { "user": { "name": "John Doe", "email": "john.doe@example.com" } } } ```
Conclusion
The synergy between CodeIgniter and GraphQL offers a powerful way to build efficient, scalable, and flexible APIs. With GraphQL, you can easily cater to the varied data requirements of your frontend applications without multiple endpoints or over-fetching data. Moreover, the static typing and introspection capabilities of GraphQL can significantly boost your API’s reliability and maintainability.
Whether you’re building a small application or an enterprise-grade system, consider leveraging the combined powers of CodeIgniter and GraphQL to ensure your APIs are up to the challenges of modern web development.
Table of Contents
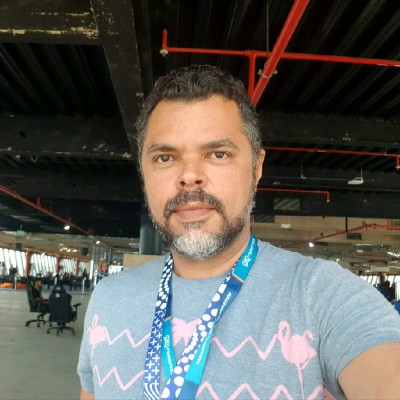
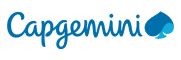