Maximizing CodeIgniter: An In-depth Guide to Libraries, Helpers, and Their Extensibility
One of the aspects that significantly contributes to CodeIgniter’s popularity is its extensibility. By leveraging CodeIgniter’s libraries and helpers, developers, including those you may choose to hire as CodeIgniter developers, can extend the functionality of this PHP framework to suit their application’s specific requirements.
This article explores how to utilize these essential features, providing a handy reference for those looking to hire CodeIgniter developers, as well as covering what these features are, how to load them, and a few examples.
Understanding CodeIgniter Libraries
In CodeIgniter, a Library refers to a class that provides a specific set of functions. Each library has a designated function and can be loaded when required, thereby enhancing the code’s efficiency. CodeIgniter comes with an array of built-in libraries, like the Form Validation Library, Session Library, and File Uploading Library. Moreover, developers can create custom libraries or extend the existing ones to suit their needs.
Loading a Library
Loading a library in CodeIgniter is a simple process. You just have to call the function `$this->load->library()`. For example, if you want to load the ‘Email’ library, you would use the following command:
```php $this->load->library('email');
You can also load multiple libraries simultaneously by passing an array of library names, as shown below:
```php $this->load->library(array('email', 'table'));
Creating Custom Libraries
Creating your custom library in CodeIgniter involves making a new file in the ‘libraries’ directory and defining your class. It’s recommended to start the class name with a capital letter.
```php class Mylibrary { public function myfunction() { // Your code here } }
Then, save the file as `Mylibrary.php` in the ‘libraries’ directory. You can load the library just like any other, with the command `$this->load->library(‘mylibrary’);`.
Diving Into CodeIgniter Helpers
Helpers in CodeIgniter, unlike libraries, are collections of procedural functions. Each helper function performs a specific task and doesn’t belong to a particular class. Some of the available built-in helpers include URL Helper, Form Helper, Text Helper, and many more.
Loading a Helper
To use a helper function in your application, you first need to load it. The syntax to load a helper is `$this->load->helper(‘name’);`. For instance, to load the ‘URL’ helper, you would use:
```php $this->load->helper('url');
To load multiple helpers, pass an array of helper names, like this:
```php $this->load->helper(array('url', 'form'));
Creating Custom Helpers
Creating a custom helper involves creating a new PHP file in the ‘helpers’ directory and defining your functions. The file name should be in lowercase and must be appended with `_helper`. For example, `my_helper.php`.
```php if (!function_exists('my_function')) { function my_function() { // Your code here } }
Then, load the helper in your application using `$this->load->helper(‘my’);`.
Extending Libraries and Helpers
One of the great things about CodeIgniter is its flexibility. You can extend its native libraries to add or override functionality.
To extend a library, create a new file in the ‘libraries’ directory, name it like the library you’re extending but prefixed with ‘MY_’ (e.g., `MY_Email.php`). Then, define your class extending the original library’s class.
```php class MY_Email extends CI_Email { public function __construct() { parent::__construct(); } // Your additional methods or overriden methods }
Similarly, you can extend a helper by placing your file into the ‘helpers’ directory, prefixing the filename with ‘MY_’ (e.g., `MY_url_helper.php`), and adding your new or overridden functions.
```php if (!function_exists('base_url')) { function base_url($uri = '') { // Your code here } }
Conclusion
CodeIgniter’s libraries and helpers are an integral part of its infrastructure, highlighting why many businesses opt to hire CodeIgniter developers. These libraries and helpers enable developers to modularize code, increase reusability, and extend the framework’s functionality. By fully understanding these aspects, developers – including those you may hire as CodeIgniter developers – can unlock the full potential of CodeIgniter, leading to more efficient, maintainable, and scalable applications. Whether it’s using built-in components or creating custom ones, the flexibility of CodeIgniter provides endless possibilities, making it a highly valuable skill set for those looking to hire CodeIgniter developers. Happy coding!
(Note: This post assumes you’re using CodeIgniter 3. If you’re using CodeIgniter 4, some practices might differ.)
Table of Contents
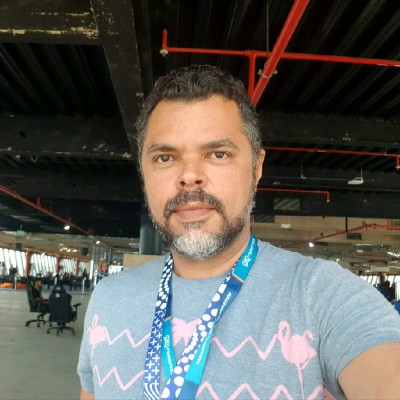
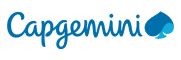