Elevate Your Web Design: Building Image Galleries with CodeIgniter
CodeIgniter, a powerful PHP framework, offers a seamless blend of simplicity, flexibility, and full-featured libraries. While CodeIgniter is primarily known for streamlining web application development, many businesses hire CodeIgniter developers specifically for its prowess in creating beautiful image galleries. In this blog post, we’ll explore the steps to craft a visually appealing image gallery using CodeIgniter, showcasing why many choose to hire CodeIgniter developers for such tasks, and providing some hands-on examples.
Table of Contents
1. Getting Started
Before you dive into the creation of an image gallery, ensure you have the following prerequisites:
– A local or remote CodeIgniter setup.
– A database to store image data (we’ll use MySQL in our examples).
– Basic knowledge of CodeIgniter’s MVC structure.
2. Setting Up the Database
We’ll start by creating a simple table in our database to store image metadata. Here’s the SQL query for the same:
```sql CREATE TABLE `gallery_images` ( `id` int(11) NOT NULL AUTO_INCREMENT, `image_name` varchar(255) NOT NULL, `title` varchar(255) DEFAULT NULL, PRIMARY KEY (`id`) ); ```
3. Setting Up CodeIgniter Configuration
Now, navigate to your `application/config/database.php` and set up your database connection:
```php $db['default'] = array( 'dsn' => '', 'hostname' => 'localhost', 'username' => 'your_username', 'password' => 'your_password', 'database' => 'your_database', // ... other configurations ... ); ```
4. Building the Model
Create a file `Gallery_model.php` in `application/models` and add the following code:
```php <?php class Gallery_model extends CI_Model { public function __construct() { $this->load->database(); } public function get_images() { $query = $this->db->get('gallery_images'); return $query->result_array(); } public function insert_image($data) { return $this->db->insert('gallery_images', $data); } } ?> ```
5. Building the Controller
Create a file `Gallery.php` in `application/controllers` and add:
```php <?php class Gallery extends CI_Controller { public function __construct() { parent::__construct(); $this->load->model('Gallery_model'); $this->load->helper('url'); } public function index() { $data['images'] = $this->Gallery_model->get_images(); $this->load->view('gallery/index', $data); } public function upload() { // handle image upload logic } } ?> ```
6. Designing the View
In `application/views`, create a new folder named `gallery` and inside, create a file `index.php`. Add:
```html <!DOCTYPE html> <html> <head> <title>CodeIgniter Image Gallery</title> <!-- Include any CSS or JS here --> </head> <body> <h1>Image Gallery</h1> <div class="image-grid"> <?php foreach ($images as $image): ?> <div class="image-container"> <img src="<?php echo base_url('uploads/' . $image['image_name']); ?>" alt="<?php echo $image['title']; ?>"/> <p><?php echo $image['title']; ?></p> </div> <?php endforeach; ?> </div> </body> </html> ```
7. Styling Your Gallery
This step is more subjective, but you might consider using a CSS grid or flexbox to align images neatly. You can also add hover effects, transitions, or lightbox libraries for a more interactive experience.
8. Uploading Images
For image uploads, you’d utilize CodeIgniter’s upload library. It simplifies the process of file validation, handling, and saving. We’ll integrate this in our `upload()` method in the `Gallery` controller.
Conclusion
With CodeIgniter’s comprehensive tools and libraries, creating an image gallery becomes a straightforward task even for those looking to hire CodeIgniter developers. This basic example can be expanded with advanced features like pagination, search, or filtering. The key is to leverage the MVC architecture effectively and make the most of the available libraries and helpers. Dive into CodeIgniter’s documentation or consult with expert CodeIgniter developers to discover more about its rich features and enhance your gallery’s functionality!
Table of Contents
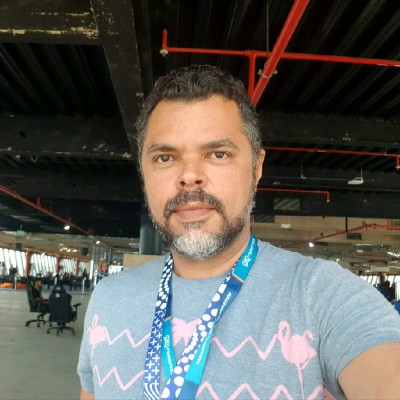
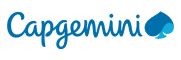