How to implement a RESTful API using CodeIgniter?
To implement a RESTful API using CodeIgniter, you can leverage its built-in features and follow RESTful principles. Here’s a step-by-step guide on how to create a RESTful API with CodeIgniter:
- Setup CodeIgniter:
First, ensure you have CodeIgniter installed and configured in your project directory. You can install it using Composer or download it directly from the official website.
- Create a Controller:
Create a new controller dedicated to handling API requests. For example, you can name it `Api.php`. In this controller, define methods corresponding to the CRUD (Create, Read, Update, Delete) operations you want to support.
- Define Routes:
Define routes in CodeIgniter’s `app/Config/Routes.php` file to map HTTP methods (GET, POST, PUT, DELETE) to the controller methods. For example:
```php $routes->resource('api/resource'); ```
This maps standard RESTful HTTP methods to the appropriate controller methods automatically.
- Implement Controller Methods:
In your `Api.php` controller, implement methods for handling API requests. These methods should perform the necessary database operations and return responses in JSON format. Here’s an example for a GET request:
```php public function show($id) { $data = $this->yourModel->getRecordById($id); if ($data) { return $this->response->setJSON($data); } else { return $this->response->setStatusCode(404)->setJSON(['error' => 'Record not found']); } } ```
- Enable CORS (Cross-Origin Resource Sharing):
If your API will be accessed by clients from different domains, configure CORS settings in CodeIgniter to allow cross-origin requests.
- Authentication and Authorization:
Implement authentication and authorization mechanisms to secure your API. You can use libraries like JWT (JSON Web Tokens) for authentication.
- Testing and Documentation:
Thoroughly test your API endpoints using tools like Postman or Insomnia. Create clear and comprehensive API documentation to help developers understand how to use your API effectively.
- Versioning:
Consider implementing versioning for your API endpoints to ensure backward compatibility as your API evolves.
- Logging and Error Handling:
Implement proper logging and error handling to track and debug issues effectively.
- Deployment:
Deploy your CodeIgniter application with the RESTful API to a web server or cloud hosting platform.
By following these steps, you can create a robust and scalable RESTful API using CodeIgniter. Remember to adhere to RESTful principles, including using appropriate HTTP methods and status codes, to make your API user-friendly and developer-friendly.
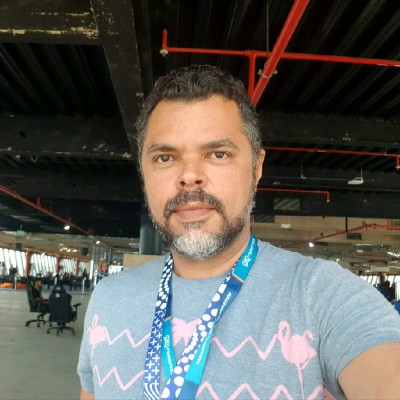
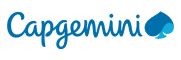