How to implement AJAX in CodeIgniter applications?
Implementing AJAX (Asynchronous JavaScript and XML) in CodeIgniter applications can enhance user interactivity by enabling dynamic content loading without the need to refresh the entire page. AJAX allows you to send and receive data from the server asynchronously, providing a smoother and more responsive user experience. Here’s how to implement AJAX in CodeIgniter applications:
- Include jQuery or JavaScript Library:
First, ensure you have a JavaScript library like jQuery included in your project. You can include it using a CDN or by downloading the library and linking to it in your HTML templates.
- Create an AJAX Request:
In your JavaScript code, create an AJAX request using the library you included. You can use functions like `$.ajax()` in jQuery to send requests to CodeIgniter controllers. Specify the URL to your CodeIgniter controller’s method, HTTP method (GET, POST, etc.), and any data you want to send.
```javascript $.ajax({ url: 'your_controller/your_method', type: 'GET', data: { param1: 'value1', param2: 'value2' }, success: function(response) { // Handle the response from the server }, error: function() { // Handle errors, if any } }); ```
- Create a Controller Method:
In your CodeIgniter application, create a controller method that handles the AJAX request. This method should perform the desired server-side operation and return a response in a suitable format, often in JSON or HTML.
```php public function your_method() { // Perform server-side logic $data = ['result' => 'Some data to return']; // Return JSON response echo json_encode($data); } ```
- Handle the Response:
In the `success` callback function of your AJAX request, handle the response from the server. You can update the HTML content of your page, manipulate DOM elements, or display the received data as needed.
- Error Handling (Optional):
Implement error handling in the `error` callback function to manage errors that may occur during the AJAX request.
With these steps, you can effectively implement AJAX in your CodeIgniter applications, enabling you to create interactive and dynamic web pages that respond to user actions without the need for full page reloads. AJAX is a powerful tool for enhancing user experiences by providing real-time updates and improving application responsiveness.
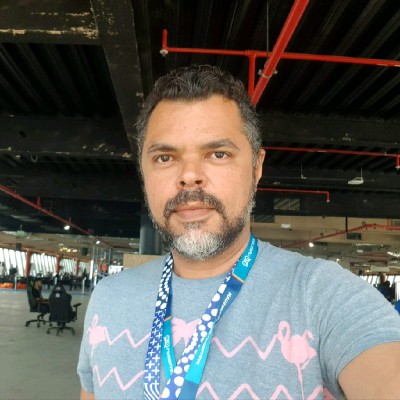
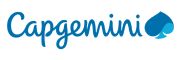