How to implement user registration and login systems in CodeIgniter?
Implementing user registration and login systems in CodeIgniter is a fundamental aspect of many web applications. Here’s a step-by-step guide on how to accomplish this:
- Database Setup:
Begin by setting up your database to store user information. Create a table, typically named “users,” with columns for user ID, username, email, password (hashed for security), and any other relevant user data.
- Controller for Registration:
Create a CodeIgniter controller, such as “Auth,” to handle registration functionality. In this controller, you’ll have methods for displaying registration forms and processing form submissions.
- Registration Form View:
Design a registration form view file that allows users to enter their registration details, including username, email, and password. Make sure to include validation rules for form fields.
- Validation and Data Insertion:
Use CodeIgniter’s form validation library to validate user input. If the data passes validation, insert it into the database after hashing the password for security.
- Controller for Login:
Create another controller method, such as “login,” for handling user login functionality. This method will display the login form and process login attempts.
- Login Form View:
Design a login form view where users can enter their credentials (username/email and password) to log in.
- Authentication:
Implement authentication logic in your login controller method. Verify user credentials against the data stored in the database. If authentication is successful, create a user session to keep the user logged in.
- Session Management:
CodeIgniter provides a session library for managing user sessions. Use this library to set and check user session variables, which determine whether a user is logged in.
- Logout Functionality:
Create a logout controller method to log users out by destroying their session data.
- Access Control:
Depending on your application’s requirements, implement access control features to restrict certain pages or actions to authenticated users only.
- Security Considerations:
Ensure that your registration and login systems are secure by using password hashing, CSRF protection, and input validation to prevent common security vulnerabilities.
- User Experience:
Enhance the user experience with helpful error messages, redirects, and user-friendly interfaces.
By following these steps and leveraging CodeIgniter’s built-in libraries and features, you can implement robust user registration and login systems in your web application. Remember to prioritize security and usability to create a seamless and secure experience for your users.
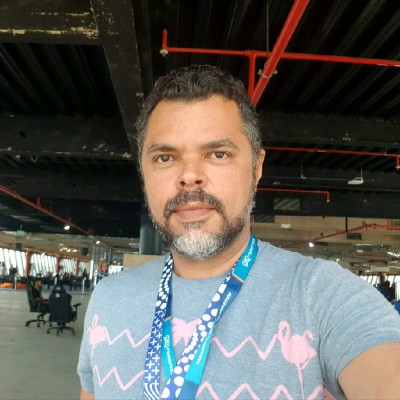
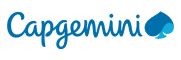