How to implement a login with Google or Facebook feature in CodeIgniter?
Implementing a login with Google or Facebook feature in a CodeIgniter application involves integrating OAuth authentication with the respective platforms. Here’s a step-by-step guide:
- Set Up OAuth Apps:
– For Google, go to the Google Developer Console (https://console.developers.google.com/).
– For Facebook, go to the Facebook for Developers website (https://developers.facebook.com/).
Create new OAuth applications for your CodeIgniter project. These apps will provide you with client IDs and secrets that you’ll need for authentication.
- Install OAuth Libraries:
Install PHP OAuth libraries that can handle OAuth2 authentication. Some popular choices include OAuth 2.0 Client by The PHP League and HybridAuth. You can use Composer to install these libraries:
``` composer require league/oauth2-client ```
- Configuration:
Configure your OAuth library with the client ID and secret obtained from Google or Facebook. Set up callback URLs for successful authentication.
- Code Implementation:
In your CodeIgniter controller, create methods to initiate the authentication process for Google and Facebook separately. For example:
– For Google:
– Redirect users to the Google OAuth URL.
– Handle the callback, exchange the authorization code for an access token, and retrieve user details.
– For Facebook:
– Redirect users to the Facebook OAuth URL.
– Handle the callback, exchange the authorization code for an access token, and retrieve user details.
- User Registration and Login:
Once you have user details from Google or Facebook, check if the user is already registered in your application. If not, create a new user record in your database with the retrieved information.
- Session Management:
Use CodeIgniter’s session library to manage user sessions. Upon successful login via Google or Facebook, set up a session for the user to maintain their login state.
- Redirect and Access Control:
After authentication, redirect the user to the appropriate page based on your application’s logic. Implement access control to secure specific routes or functionalities.
- Logout:
Provide a logout option that clears the user’s session and logs them out of your application.
- Testing:
Thoroughly test the login with Google or Facebook feature to ensure that user data is handled securely and that the authentication flow works as expected.
By following these steps, you can successfully implement a login with Google or Facebook feature in your CodeIgniter application, providing users with the convenience of using their existing social media accounts for authentication.
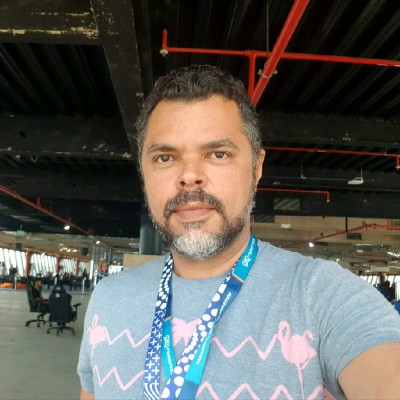
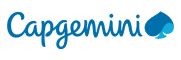