CodeIgniter and IoT: Connecting Your App with Internet of Things Devices
In the digital age, the Internet of Things (IoT) is revolutionizing how we interact with the physical world. From smart homes to industrial automation, IoT devices are becoming integral parts of our daily lives. As developers, integrating these devices with web applications can open new possibilities and enhance user experiences. In this blog, we’ll explore how to use CodeIgniter, a popular PHP framework, to connect your app with IoT devices.
Understanding the Basics of IoT
IoT refers to the interconnection of everyday devices via the internet, enabling them to send and receive data. These devices can range from simple sensors and actuators to complex systems like smart thermostats and industrial machines. The key components of an IoT system include:
- Sensors and Actuators: Devices that collect data or perform actions.
- Connectivity: Methods to connect devices, such as Wi-Fi, Bluetooth, or cellular networks.
- Data Processing: Analysis and processing of collected data.
- User Interface: Applications or dashboards for monitoring and control.
Why Use CodeIgniter for IoT?
CodeIgniter is a lightweight PHP framework known for its speed and simplicity. It provides a robust platform for building web applications, making it an excellent choice for developing IoT dashboards and control panels. Key advantages include:
- MVC Architecture: CodeIgniter’s Model-View-Controller (MVC) structure helps in organizing code efficiently, making it easier to manage IoT data and user interfaces.
- Built-in Libraries: It offers built-in libraries for form validation, session management, and more, simplifying development.
- Security Features: CodeIgniter includes security measures to protect your application from common web vulnerabilities.
Setting Up CodeIgniter for IoT Integration
1. Installing CodeIgniter
To get started, download and set up CodeIgniter. Ensure your server meets the necessary requirements, such as PHP 7.4+ and a compatible web server (Apache or Nginx).
```bash # Navigate to your web directory cd /var/www/html # Download CodeIgniter wget https://codeigniter.com/download # Extract the downloaded file unzip CodeIgniter-*.zip ```
2. Configuring Routes and Controllers
In CodeIgniter, routes define URL patterns and their corresponding controllers. For an IoT application, you might have routes for viewing device data, controlling devices, etc.
```php // In application/config/routes.php $route['default_controller'] = 'Devices'; $route['devices/view'] = 'Devices/view'; $route['devices/control'] = 'Devices/control'; ```
3. Creating Models for IoT Data
Models in CodeIgniter handle data operations. For instance, you can create a model to interact with a database that stores sensor readings.
```php // In application/models/Device_model.php class Device_model extends CI_Model { public function get_device_data($device_id) { $query = $this->db->get_where('devices', array('id' => $device_id)); return $query->row_array(); } } ```
4. Developing Controllers for IoT Actions
Controllers process requests and display the appropriate response. For IoT, this might include fetching data from sensors or sending commands to actuators.
```php // In application/controllers/Devices.php class Devices extends CI_Controller { public function view($device_id) { $data['device'] = $this->Device_model->get_device_data($device_id); $this->load->view('devices/view', $data); } public function control($device_id, $action) { // Code to send control signals to the device } } ```
5. Designing Views for Data Display
Views are used to display data to the user. You can create a simple dashboard to show device status, control options, and more.
```php <!-- In application/views/devices/view.php --> <h1>Device Status</h1> <p>Temperature: <?php echo $device['temperature']; ?>°C</p> <p>Humidity: <?php echo $device['humidity']; ?>%</p> <a href="/devices/control/<?php echo $device['id']; ?>/turn_on">Turn On</a> <a href="/devices/control/<?php echo $device['id']; ?>/turn_off">Turn Off</a> ```
Security Considerations
When dealing with IoT, security is paramount. Ensure that:
- All communications between the web application and IoT devices are encrypted.
- Implement user authentication and authorization to prevent unauthorized access.
- Regularly update your CodeIgniter application and IoT device firmware.
Conclusion
Integrating CodeIgniter with IoT devices can significantly enhance the functionality of your web applications. Whether you’re building smart home systems, industrial monitoring platforms, or anything in between, CodeIgniter provides a solid foundation for IoT development. Start experimenting with your IoT projects today and unlock the endless possibilities of connected devices!
Further Reading
Table of Contents
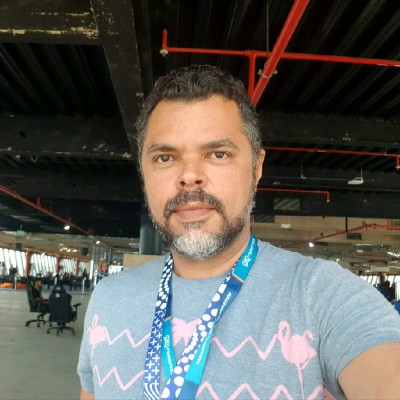
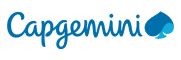