Crafting Secure APIs: Integrate JWT with CodeIgniter the Right Way
In today’s world, security has become paramount, especially when dealing with sensitive data over the internet. One of the most popular frameworks in the PHP community, CodeIgniter, offers simplicity and speed in creating web applications. However, when it comes to creating APIs with CodeIgniter, ensuring security can be a bit of a challenge.
One popular method to ensure the security of API endpoints is through the use of JSON Web Tokens (JWT). JWTs provide a way to encode and verify data passed between the client and the server.
In this article, we’ll delve deep into how you can integrate JWT with CodeIgniter to secure your API endpoints.
1. What is a JSON Web Token (JWT)?
A JWT is a compact, URL-safe token format that is used for securely transmitting information between parties. It’s divided into three parts:
- Header: Contains token type and hashing algorithm.
- Payload: Contains the claims or the data we want to store in the token.
- Signature: Used to verify if the message wasn’t changed along the way.
Combining these three gives us a JWT, which looks something like: `xxxxx.yyyyy.zzzzz`
2. Setting up CodeIgniter
Before we dive into JWT, let’s set up a basic CodeIgniter project:
- Download and install CodeIgniter from the official website.
- Configure your database in `application/config/database.php`.
- Create an API controller in `application/controllers/ApiController.php`.
3. Integrating JWT with CodeIgniter
For simplicity, we’ll use the PHP-JWT library by Firebase.
- Install it using Composer:
``` composer require firebase/php-jwt ```
- In your `ApiController`, include the JWT library:
```php use Firebase\JWT\JWT; ```
4. Generating a Token
To create a JWT, you need a secret key and some data (or claims).
```php class ApiController extends CI_Controller { private $secretKey = "yourSecretKey"; public function generate_token() { $tokenId = base64_encode(mcrypt_create_iv(32)); $issuedAt = time(); $notBefore = $issuedAt + 10; // Adding 10 seconds $expire = $notBefore + 60; // Adding 60 seconds $serverName = 'yourDomainName.com'; $data = [ 'iat' => $issuedAt, 'jti' => $tokenId, 'iss' => $serverName, 'nbf' => $notBefore, 'exp' => $expire, 'data' => [ // Data related to the signer user 'userId' => 'yourUserId', 'userName' => 'yourUserName' ] ]; $jwt = JWT::encode( $data, $this->secretKey, 'HS512' ); $response = ['token' => $jwt]; echo json_encode($response); } } ```
5. Validating the Token
Before granting access to your API’s secure endpoint, you need to validate the token:
```php public function validate_token($token) { try { $decoded = JWT::decode($token, $this->secretKey, ['HS512']); return $decoded->data; } catch (Exception $e) { // Token is invalid // Send the user an error message $response = ['status' => 'error', 'message' => 'Token is invalid']; echo json_encode($response); exit; } } ```
6. Securing an API Endpoint
With the above methods in place, securing an endpoint becomes easy. For instance, if you have a method to fetch user details:
```php public function get_user_details() { $headers = $this->input->request_headers(); if (isset($headers['Authorization'])) { $token = $headers['Authorization']; $user_data = $this->validate_token($token); // If token is valid, fetch user data from database using $user_data->userId // ... your code ... } else { $response = ['status' => 'error', 'message' => 'Missing token']; echo json_encode($response); } } ```
Conclusion
Securing your API endpoints is crucial, especially in a world where data breaches are commonplace. Integrating JWT with CodeIgniter offers a robust solution, allowing you to ensure the authenticity and integrity of the data exchanged between clients and servers. With the above guide, you should be well on your way to creating a more secure API with CodeIgniter using JSON Web Tokens.
Table of Contents
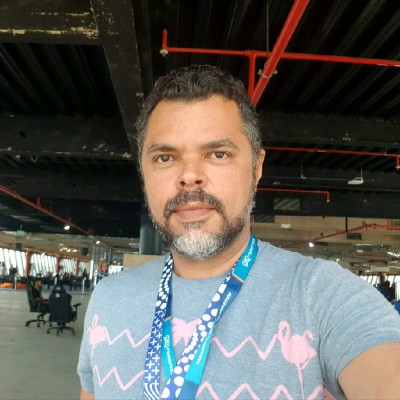
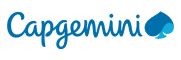